You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Once I include the polygon into a polygon set data, however, it creates an additional unwanted point (8,8) from (8,10)->(8,2). Even boost::polygon::simplify leaves this point. Is that the expected behavior?
ps.get(polygons); function applies a union operation to the polygons. This is by definition. If you add overlapping polygons and call get function you will get merged results, it won't match your original input.
I want to represent a donut shape with
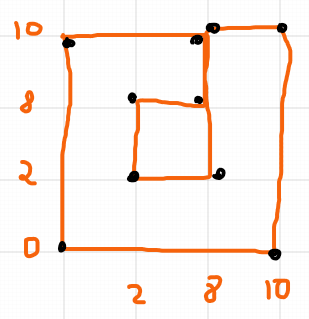
boost::polygon
, such as the figure below, with the following points:Once I include the polygon into a polygon set data, however, it creates an additional unwanted point
(8,8)
from(8,10)->(8,2)
. Evenboost::polygon::simplify
leaves this point. Is that the expected behavior?The text was updated successfully, but these errors were encountered: