diff --git a/README.md b/README.md
index a0e2f0f2..988a288d 100644
--- a/README.md
+++ b/README.md
@@ -551,6 +551,69 @@ File content before and after merge:
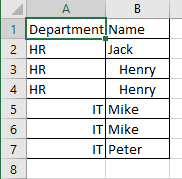
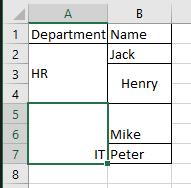
+#### 13. Skip null values
+
+Default behaviour:
+
+```csharp
+DataTable dt = new DataTable();
+
+/* ... */
+
+DataRow dr = dt.NewRow();
+
+dr["Name1"] = "Somebody once";
+dr["Name2"] = null;
+dr["Name3"] = "told me.";
+
+dt.Rows.Add(dr);
+
+MiniExcel.SaveAs(@"C:\temp\Book1.xlsx", dt);
+```
+
+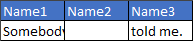
+
+```xml
+
+
+ Somebody once
+
+
+
+
+
+ told me.
+
+
+```
+
+Result using new option:
+
+```csharp
+OpenXmlConfiguration configuration = new OpenXmlConfiguration()
+{
+ WriteNullValues = false // Default value is true.
+};
+
+MiniExcel.SaveAs(@"C:\temp\Book1.xlsx", dt, configuration: configuration);
+```
+
+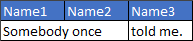
+
+
+```xml
+
+
+ Somebody once
+
+
+
+ told me.
+
+
+```
+
+Works for null and DBNull values.
### Fill Data To Excel Template
diff --git a/docs/README.md b/docs/README.md
index b1c6110e..58205e37 100644
--- a/docs/README.md
+++ b/docs/README.md
@@ -22,6 +22,9 @@
---
+### 1.30.4
+- [New] Support skipping null values when writing to Excel (via @0MG-DEN)
+
### 1.30.3
- [New] support if/else statements inside cell (via @eynarhaji)
diff --git a/src/MiniExcel/OpenXml/ExcelOpenXmlSheetWriter.cs b/src/MiniExcel/OpenXml/ExcelOpenXmlSheetWriter.cs
index f7c6a665..0ed7bf96 100644
--- a/src/MiniExcel/OpenXml/ExcelOpenXmlSheetWriter.cs
+++ b/src/MiniExcel/OpenXml/ExcelOpenXmlSheetWriter.cs
@@ -395,14 +395,19 @@ private void GenerateSheetByColumnInfo(MiniExcelStreamWriter writer, IEnumera
private void WriteCell(MiniExcelStreamWriter writer, int rowIndex, int cellIndex, object value, ExcelColumnInfo p)
{
- var v = string.Empty;
- var t = "str";
+ var columname = ExcelOpenXmlUtils.ConvertXyToCell(cellIndex, rowIndex);
var s = "2";
- if (value == null)
+
+ if (!_configuration.WriteNullValues && (value is null || value is DBNull))
{
- v = "";
+ writer.Write($"");
+ return;
}
- else if (value is string str)
+
+ var v = string.Empty;
+ var t = "str";
+
+ if (value is string str)
{
v = ExcelOpenXmlUtils.EncodeXML(str);
}
@@ -531,7 +536,6 @@ private void WriteCell(MiniExcelStreamWriter writer, int rowIndex, int cellIndex
}
}
- var columname = ExcelOpenXmlUtils.ConvertXyToCell(cellIndex, rowIndex);
if (v != null && (v.StartsWith(" ", StringComparison.Ordinal) || v.EndsWith(" ", StringComparison.Ordinal))) /*Prefix and suffix blank space will lost after SaveAs #294*/
writer.Write($"{v}");
else
diff --git a/src/MiniExcel/OpenXml/OpenXmlConfiguration.cs b/src/MiniExcel/OpenXml/OpenXmlConfiguration.cs
index df874b87..26f29645 100644
--- a/src/MiniExcel/OpenXml/OpenXmlConfiguration.cs
+++ b/src/MiniExcel/OpenXml/OpenXmlConfiguration.cs
@@ -16,6 +16,7 @@ public class OpenXmlConfiguration : Configuration
public bool AutoFilter { get; set; } = true;
public bool EnableConvertByteArray { get; set; } = true;
public bool IgnoreTemplateParameterMissing { get; set; } = true;
+ public bool WriteNullValues { get; set; } = true;
public bool EnableSharedStringCache { get; set; } = true;
public long SharedStringCacheSize { get; set; } = 5 * 1024 * 1024;
}