-
Notifications
You must be signed in to change notification settings - Fork 5
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Address.ParseAddress function failed #99
Comments
Usage:
Bech32 class
CKBAddress
|
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here is my testing data:
When I test some code, the ParseAddress always failed.
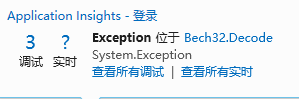
It always throw exception of
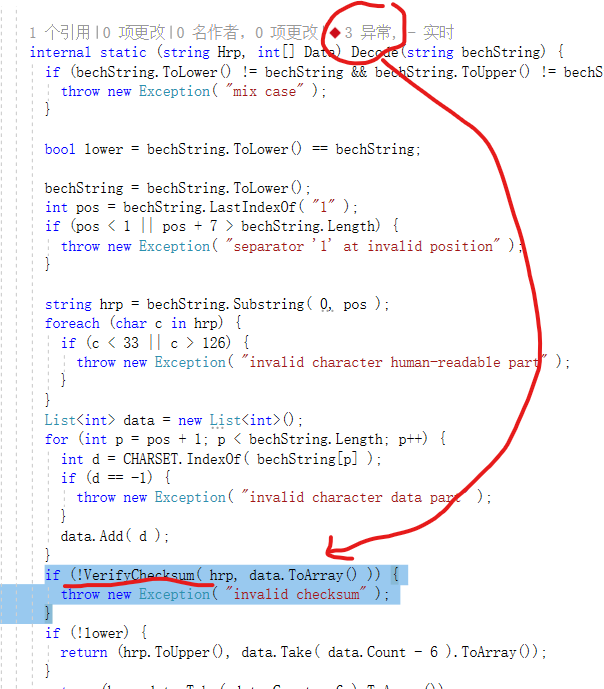
invalid checksum
The text was updated successfully, but these errors were encountered: