-
Notifications
You must be signed in to change notification settings - Fork 10
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Add support for custom texture #29
base: dev
Are you sure you want to change the base?
Conversation
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I think this is a potentially great new feature.
First, the small things.
Your way to create the default textures using Images instead of RenderTextures is far better than the current one. If you wanted to isolate that in a separate PR I would merge it immediatlely. Also, I would have to re-read the problems with the crash and the issue in SFML, but its probable that the change would fix it, for what I remember.
Now, about the real proposal.
Adding a new attribute to the light is a big change and, following the current philosophy of the project, there are several important things to take into account.
- Conceptually speaking, the light should only have one texture (even though behind there were two, as right now). So we would need to think of a design where there are not
setLightFadeTexture
andsetLightPlainTexture
but onlysetTexture
.- This restriction could also be the answer to the problem of the 1px transparent border: the setter could assign the given texture to the
m_lightTexturePlain
and then make a processed copy to makem_lightTextureFade
(applying the fade effect and the transparent border). However, we would need to be very careful managing this inner resource...
- This restriction could also be the answer to the problem of the 1px transparent border: the setter could assign the given texture to the
- There would have to be functions to customize the texture. The bar is set by the texture of
LightingArea
, so the minimum function I would require before release would besetTextureRect
.- This reminds me that I have to add more texture related functions like
setTextureRotation(float)
,setTextureRepeated(bool)
. Not urgent.
- This reminds me that I have to add more texture related functions like
- The texture attribute should belong to the parent class, LightSource and not be specific to RadialLight. I wouldn't include it in a new release before texture was implemented in DirectedLight.
As those are quite a few changes I would even have a separate branch to work on it in the main repo.
Thanks!
Some notes: |
Maybe we can put a setTexture(sf::Texture* texture) and if argument is nullptr, the default one would be taken.
no problem 👍
Mmh I wanted to do a all in one pr, but if you confirm to me that you want another pr, yeah I can.
Maybe we can add a flag : bool m_customTexture
I don't like the idea of copying texture, imagine that for 100 lights the same texture is copied again for all of them. I don't think this is memory friendly.
Yep I agree with that, this can also lead to rectangle RadialLight for more user customisation
I would like to add a setBlendMode too, this can lead to fully colored light texture, again for more user customisation
Yep agree with that |
OK, I like this idea better.
There is no hurry, we can do all this in the same pr. If that's easier for you, no problem :D
I was thinking to have an inner static caché of textures void RadialLight::setTexture(sf::Texture* texture){
if(texture == nullptr){
// ...
}
m_lightTexturePlain = texture;
auto cachedFadeTextureIter = s_fadedTexturesCache.find(texture);
if(cachedFadeTextureIter != s_fadedTexturesCache.end()){
m_lightTextureFade = *cachedFadeTextureIter;
}else{
m_lightTextureFade = makeFadeTexture(texture);
s_fadedTexturesCache[texture] = m_lightTextureFade;
}
}
The problem I see with this is that, as a user, it makes sense that the texture and the fade flag can interact:
At least, this is the behavior I would expect as a user.
Yes, why not. Only that we would have to be careful on how this would interact with a LightingArea. Side note: I'm sure that all this could be easily solved by shaders. The day we can get a contributors that knows GLSL this is going to have a huge revamp 😂 Edit: minor change in snippet |
Mmmh I agree, shaders will be so good in this case x), I think I can try some GLSL this should not be too hard 😃 |
I just found an interesting repository with a similar project: |
Hello, thank you for the shoutout ! I started working on my own light system due to specific needs i had regarding functionalities. it seems that my version is slightly more complete than yours, but also has a completely different approach and is more geared towards scenes with high numbers of lights. I haven't had time to work on it due to other projects being in the pipelines so the repo might appear dead. I have a big number of things i want to add to it however, including optimization. While i am not interested in actively taking part in the development of Candle due to having my own system at the moment, i'd be happy to discuss your objectives with your system (maybe see if my version has everything you need ?) and eventually provide help or technical assistance. If not, and if you end up reusing some of my ideas or code, i'd like to at least be credited in your repository. |
Thank you, @DaiMysha ! In case I take some non-trivial concept from your system, I'll credit you in the category Having a look at your project, I think that the biggest difference is that Candle is not properly a lighting system, but needs the user to build their own. If you wanted a scene with lots of lights, with Candle you'd have to manage which ones get updated and which one do not, while your system manages that semi-automatically, I think. I will think about this for future versions... Anyways, thanks again ❤️ . If there's anything I wanted to discuss about this, I'll open a specific issue, to avoid bloating this thread. |
This is what I love with Candle, I can simply build a framework/engine around the light system :) I will check the code from the mentioned repo and see if I can try something, thanks ! |
There must be a way to change that value... I guess from the shader it would be possible(?) |
Hi! A note of interest in this regard I hope: Another project of great interest, that I believe to be the Light System forerunner in the SFML ecosystem, is LTBL2. You may already know it. Although old it also proved to be very powerful. It is a pity that the great @222364 has not shown signs of life for such a long time... Best wishes for Candle! DJuego |
Thanks, DJuego! |
I tested a bit with GLSL, but It's hard to find something that work.
If someone want to help (in fact I don't really have the time to work with GLSL). |
I see... well, thanks for the attempt, @JonathSpirit . I'm gonna move this to another issue, keep the approach you want for this specific issue with everything we talked about in mind. I wish there were more clear tutorials for SFML+GLSL. |
I don't really like that we have to manage textures resource, maybe we can have a special structure like this :
And have a function for light texture loading :
This let the user the responsibility to manage memory resource. The last commit that I push is here to show an implementation with a std::map and std::pair (for the plain/fade texture) |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Mmm I would not add a new structure to store light textures and let the user manage it. In part because if Candle uses shaders in the future, that could cause compatibility problems between Candle versions (as the LightTexture struct wouldn't be needed once shaders enter), so we have to keep the user managing a single texture resource. The idea is to keep the API as robust as possible.
Your implementation in the last commit looks like it should work fine! Have you tried it? I guess that the memory could be a problem if you used a lot of different light textures, but there should be no problem (in that sense) using lots of lights with a few textures...
Let me know if you test it.
Thank you!
Right now I'm not sure but maybe the problem in directed light is that the |
Add a cleanupTexture(sf::texture* texture) |
The current status of this pr : I tried to implement textures into DirectedLight but I can't find a way for this to work (a bit lost on that). But overall, this work well on RadialLight.
I do not see how setTextureRect can be implemented here, the texture is bound to every points of the polygon, I think that if we want to customise the texture rect, we have to use shaders. Maybe I shouldn't have tried to implement a new feature but more to focus on optimization/cleaning because it becomes a bit complicated to navigate through the code.
I'm gonna open a new pull request just for the texture modification cause It can fix some weird issues and for now I will leave this PR in this state because I think it would need a bit more of a solid base. |
Hi, @JonathSpirit.
You are probably right. That's something I was afraid of when I first started working with lighting, and finally the moment has come! The last time I checked the code from the repository of DaiMysha, the shader looked like something feasible to implement in Candle. I'll be updating. |
(note that this is a in progress pr and need testing)
Add the possibility to change the light texture with a custom one.
Notable change (RadialLight) :
calculation of the pixels in initializeTextures is now smoother and math friendly
in line 285 of RadialLight.cpp, the maximum range distance is reduced too avoid too far vertex for the polygon :
Known problem :
When the texture have non-transparent pixels at his border, a bright glitch can appear. This is due to the coloured (in this example white) vertex that are too far from the texture bound, this will cause OpenGL to draw some weird pixel in order to fill the entire polygon.
To avoid that, I was thinking about 3 solutions :
Screenshots/GIFs :
no border :
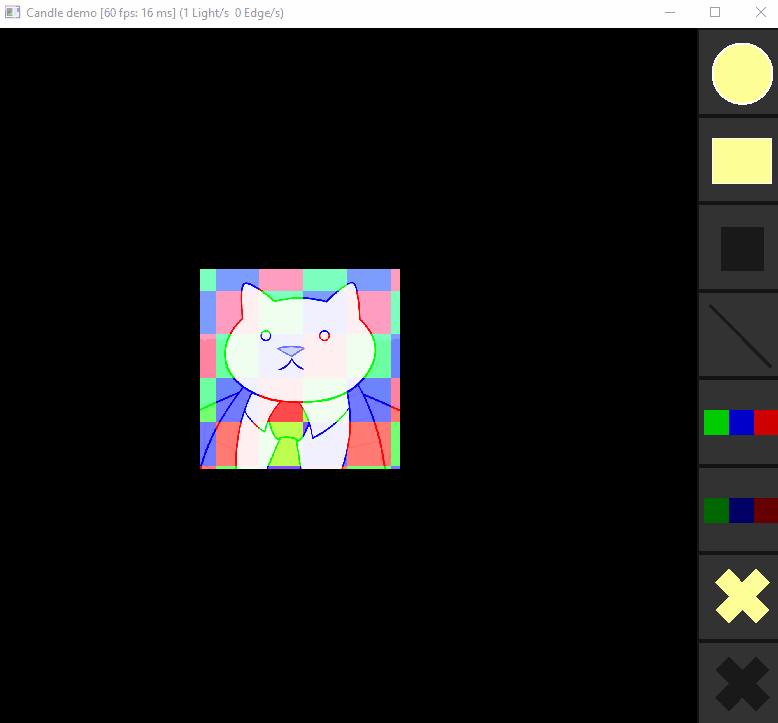
with transparent border :
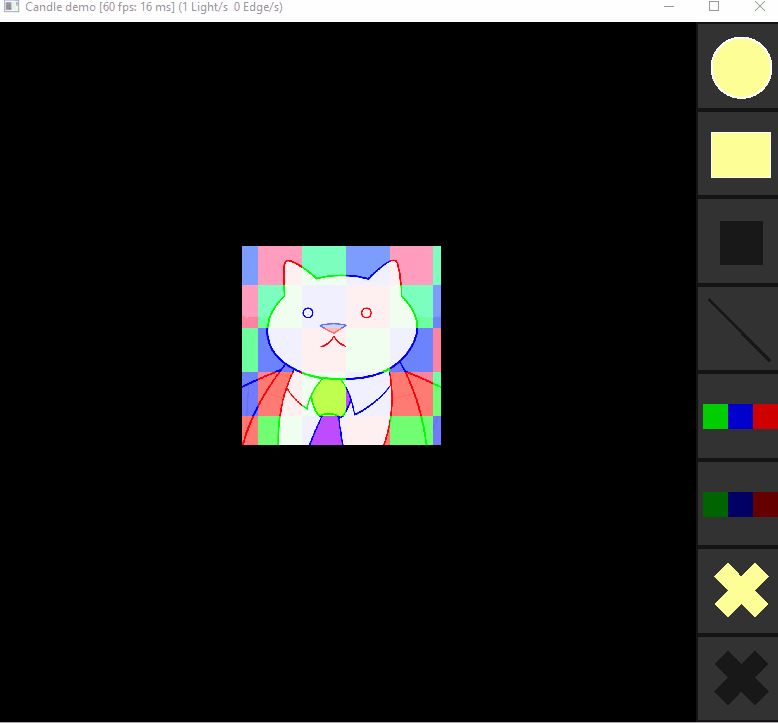
How to test (what I do) :
in the demo.cpp file
I added a global variable :
At line 369 :
In the main :
I'm open to suggestion, fix, critic etc.... 😃