Mk ble uart bug fixes with tx fifo enhancements #147
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
While using this board support package for a project, I ran some tests using a modified version of the bleuart sketch and the Adafruit Bluefruit LE Connect app on my Samsung tablet, and found some problems with the BLEUart service:
With TX buffering turned off and sending more than 20 characters from the Serial Monitor, only upto 20 would be transmitted, even the bleuart.write() function indicated all of them had been sent.
With TX buffering turned off and sending more than 20 characters from the Serial Monitor, not all them would be transmitted, even the bleuart.write() function indicated all of them had been sent. Moreover, the ones that did get sent would be sent out-of-order somewhere past the 20th character.
The TX buffer, when enabled, was set to a fixed size not settable by the user.
Here are some screenshots from the Arduino Serial Monitor and my Android tablet illustrating the problems.
In this case, 123456789012345678901234567890 (with a LF terminator) was sent. Notice how they are out of order after the 20th character ('0', or 0x30) was sent.
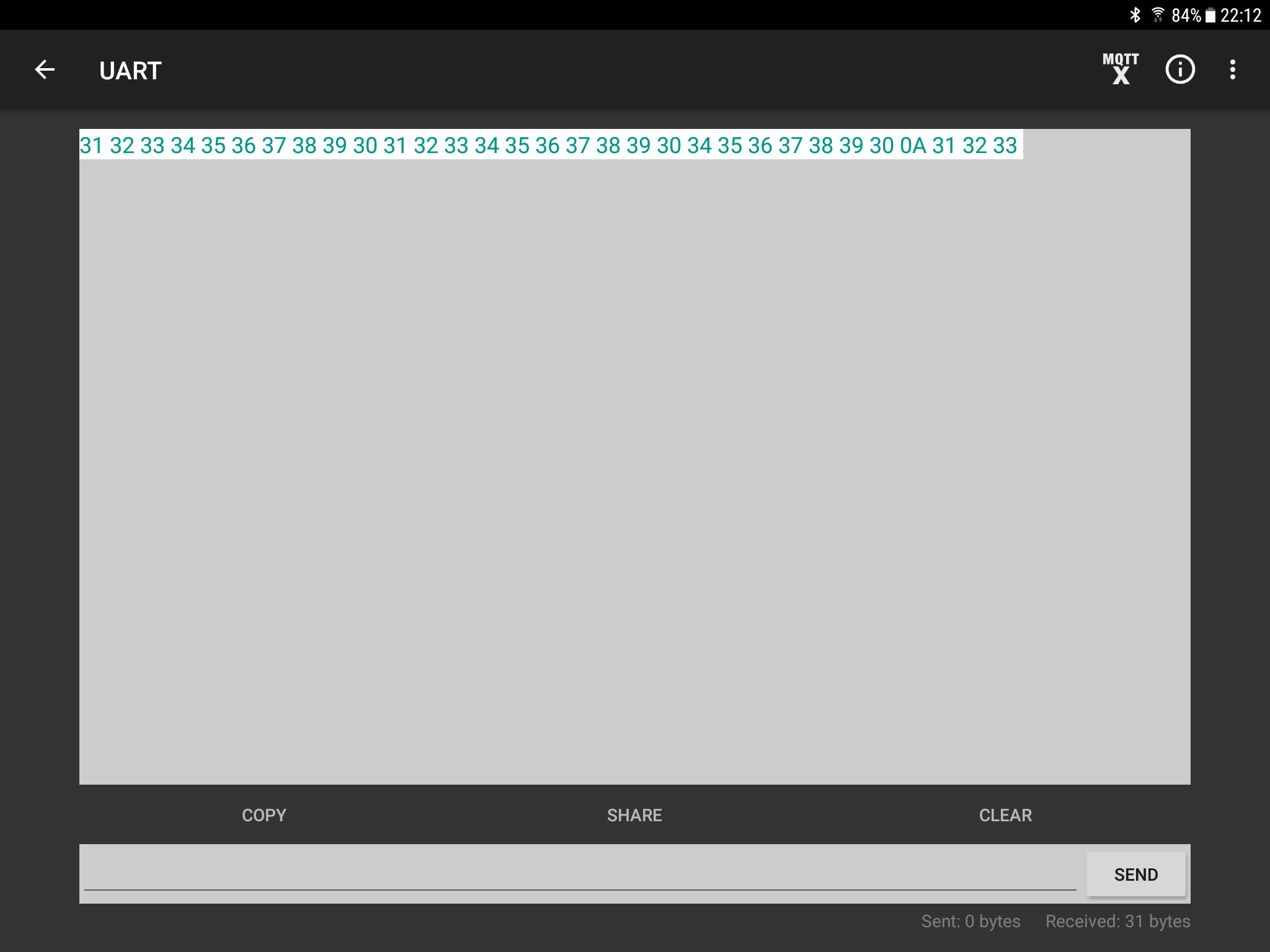
In this case, ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789abcdefghijklmnopqrstuvwxyz (with a LF terminator) was sent. Notice how they are out of order after the 20th character ('T', or 0x54) was sent.
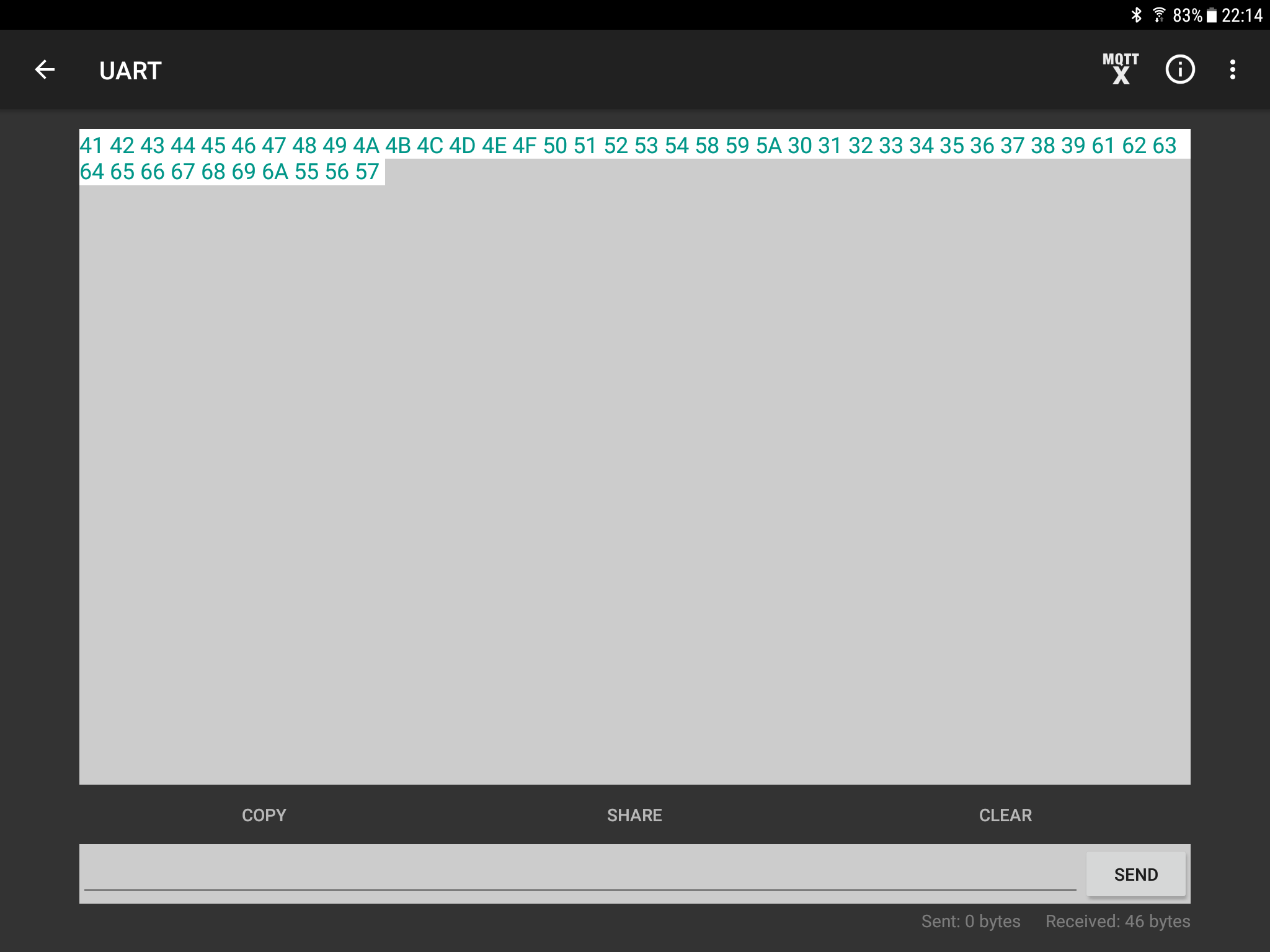
Here, the Serial Monitor reports all characters (31 for 123456789012345678901234567890, 63 for ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789abcdefghijklmnopqrstuvwxyz) were sent, even though they were not.
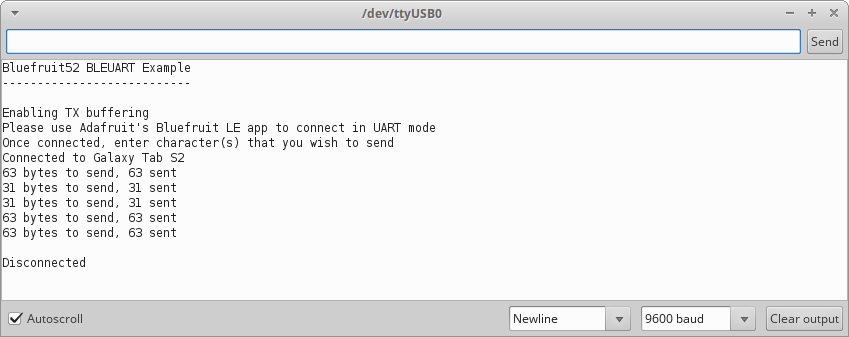
Within my development branch (mk_BLE_UART_bug_fixes_with_TX_FIFO_enhancements), I have attempted to fix all three of these problems. I have run some tests with these changes, and thus far all 3 problems have been corrected.
Here are some screenshots from the Arduino Serial Monitor and my Android tablet illustrating the same tests after the fixes.
In these test cases, the TX buffer is turned off.
Only 20 characters, as expected, were sent.
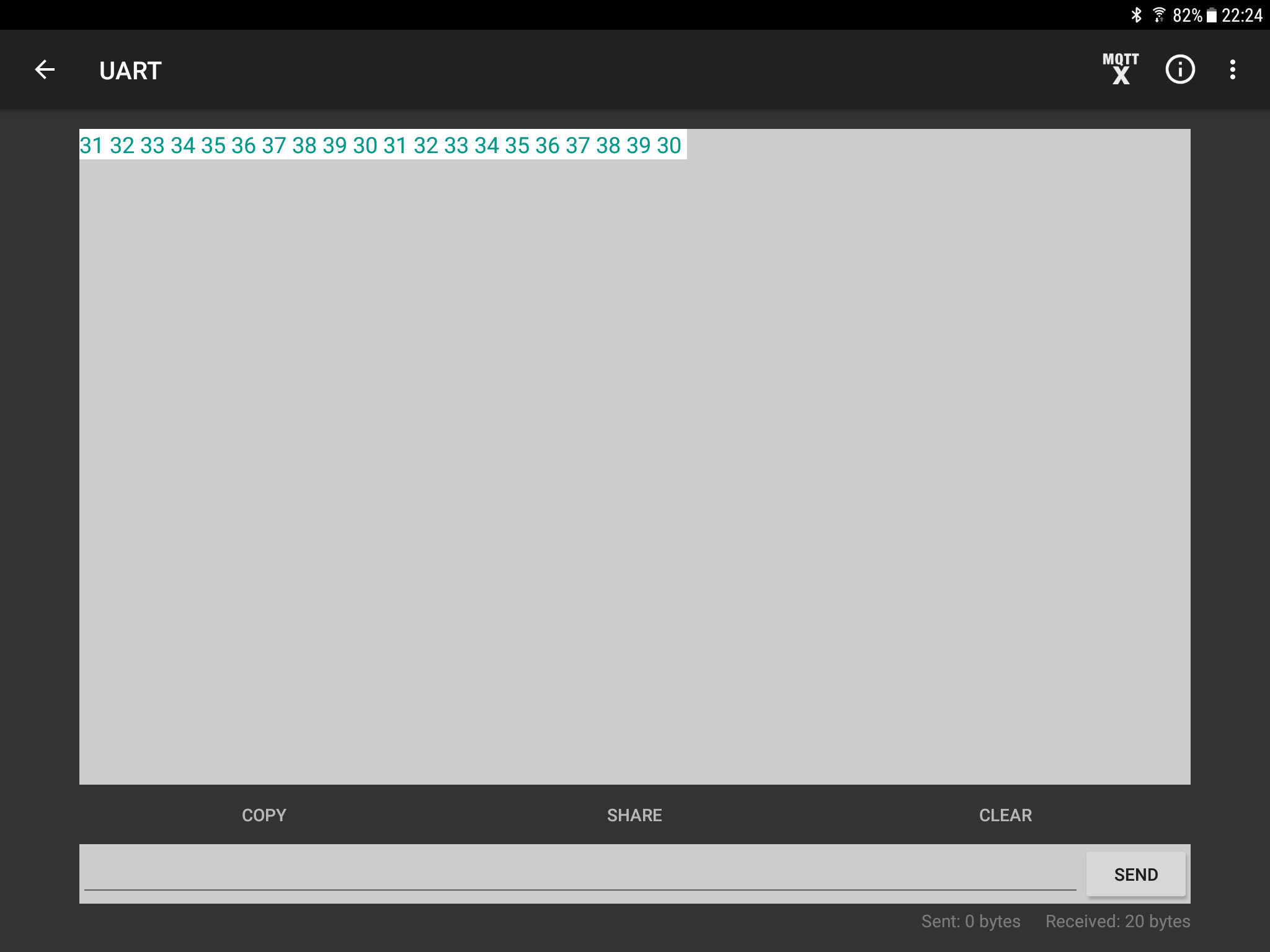
Again, only 20 characters, as expected, were sent.
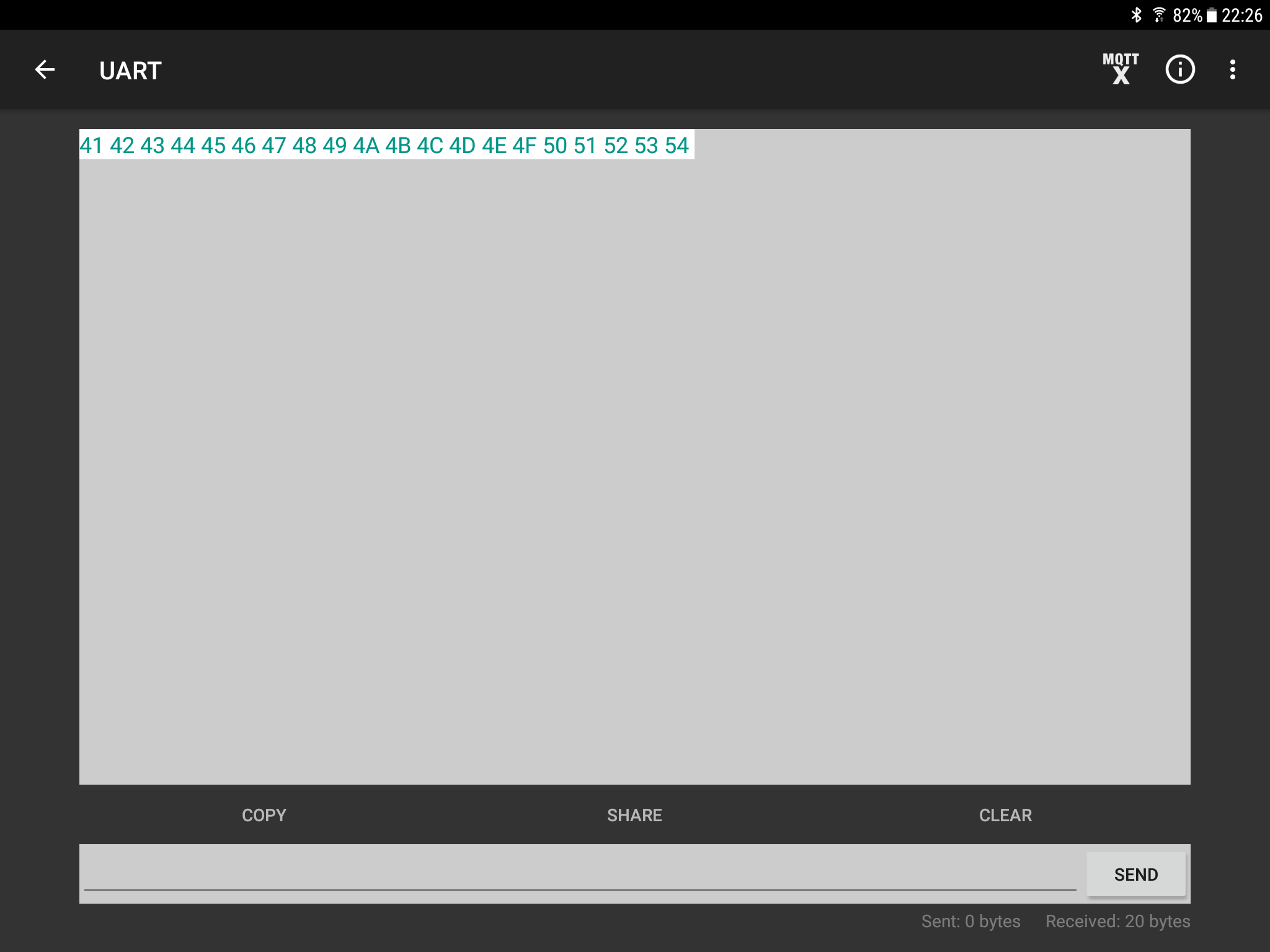
Notice how bleuart.write() now returns the actual number of characters sent.
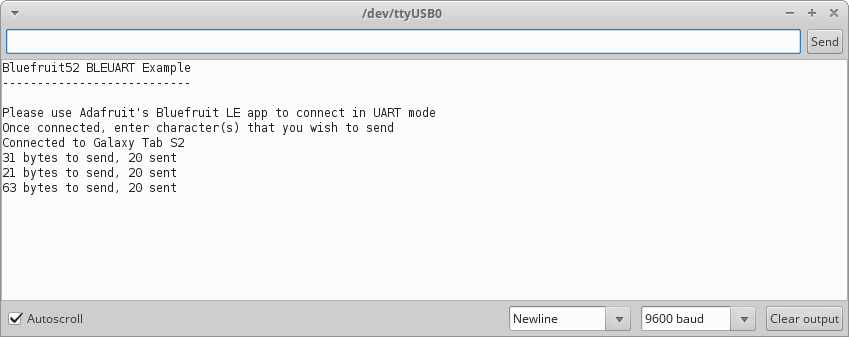
In these test cases, the TX buffer is turned on.
21 characters (all of them) were sent.
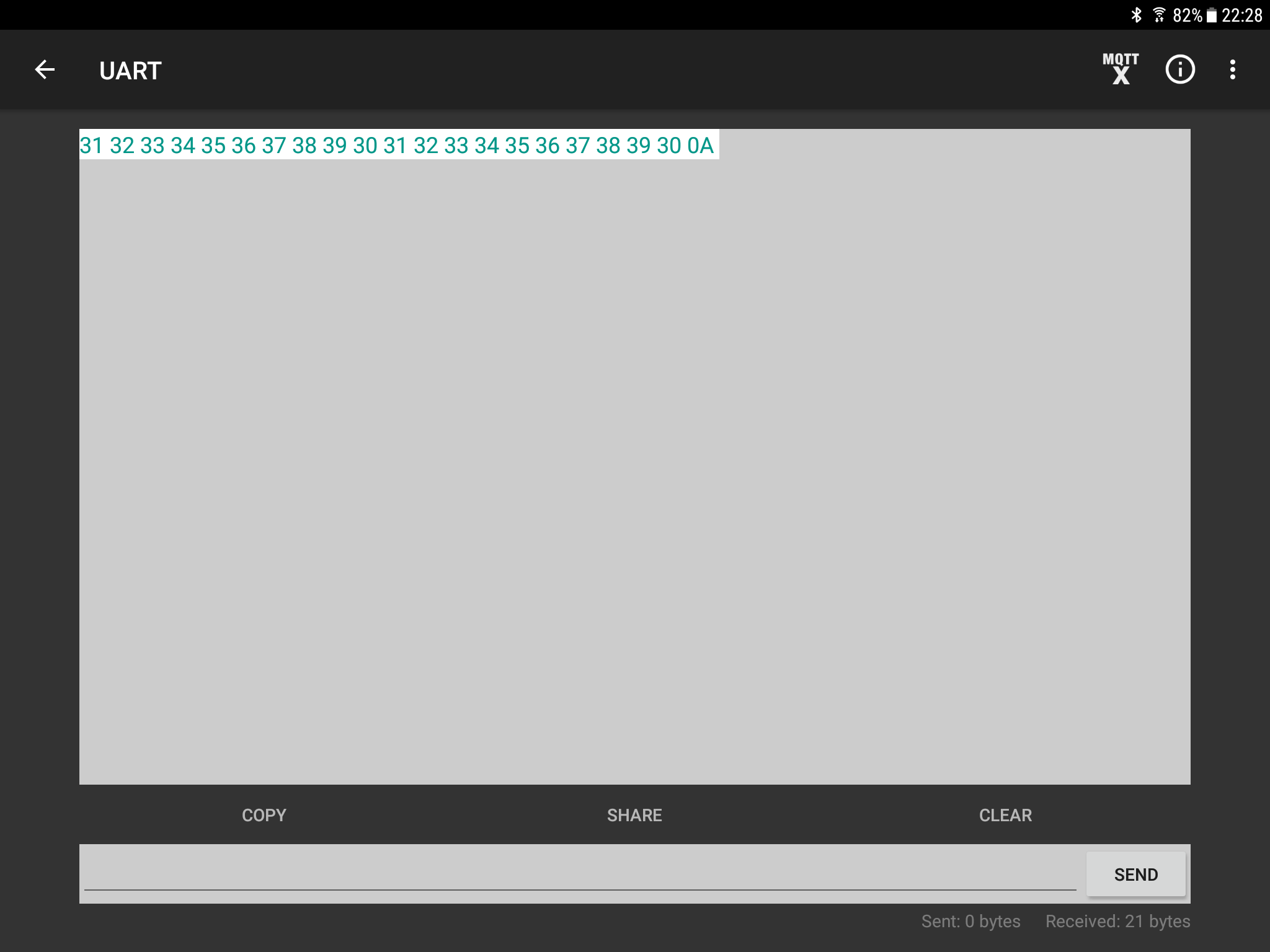
63 characters (all of them) were sent.
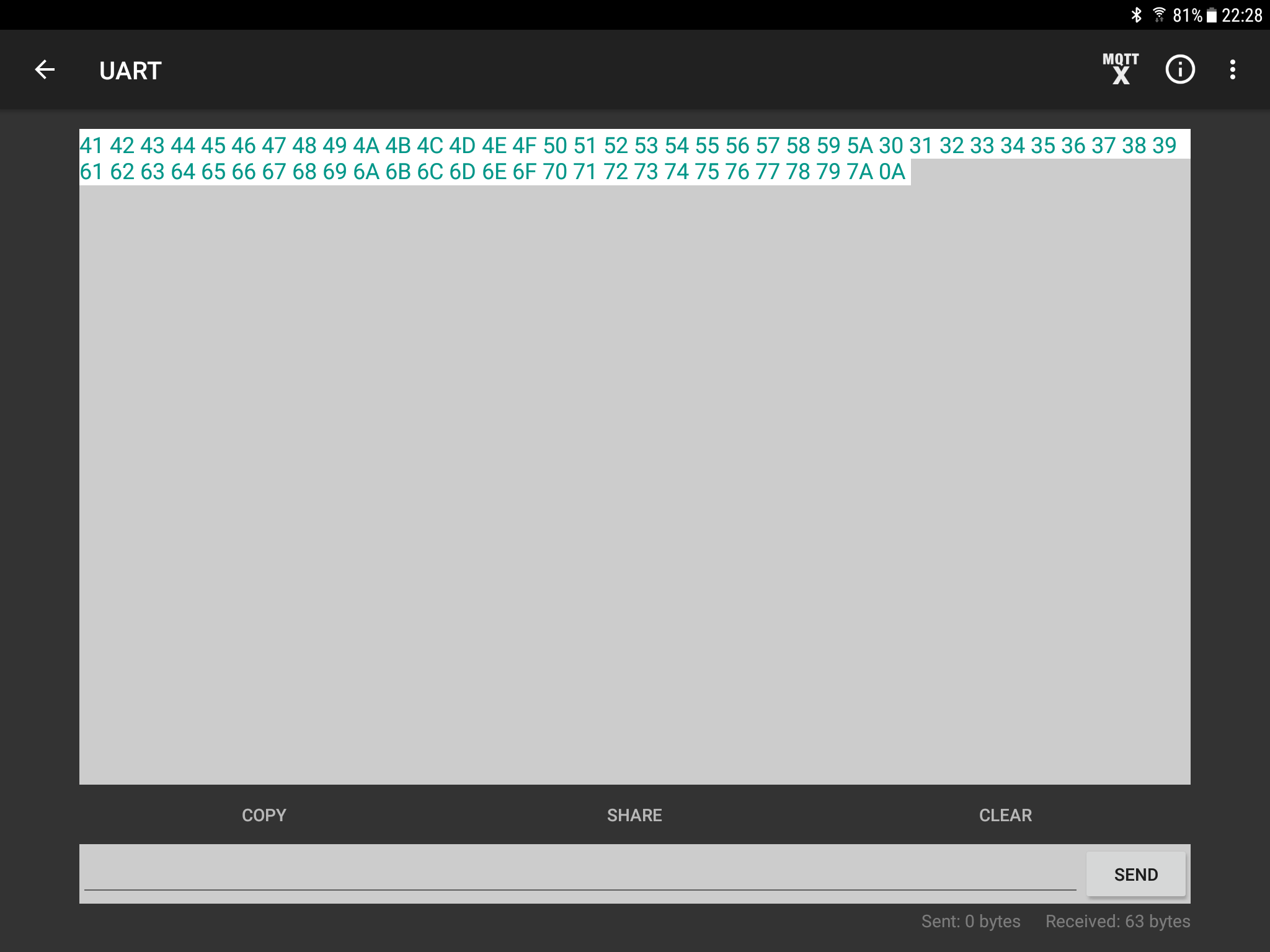
Again, bleuart.write() now returns the actual number of characters sent.
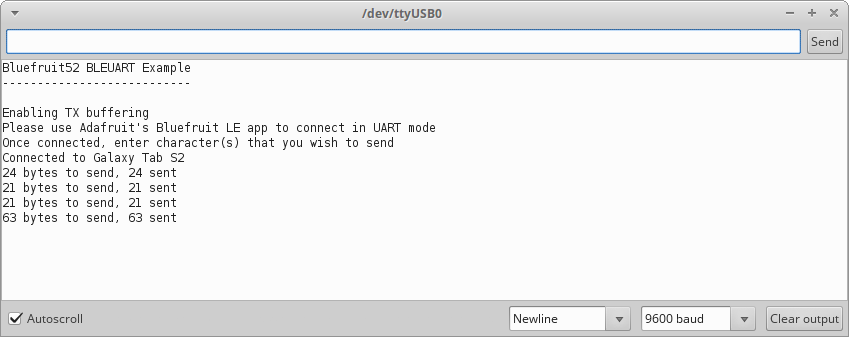
Here the modified version of the bleuart sketch I used for testing:
Please take a look at these changes, and if they seem good, please incorporate them in the board support package.
Thanks for your help
Michael Kirkhart