-
Notifications
You must be signed in to change notification settings - Fork 4.1k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Browse files
Browse the repository at this point in the history
## What <!-- * Describe what the change is solving. Link all GitHub issues related to this change. --> Adds git commit info to the metadata file during upload. 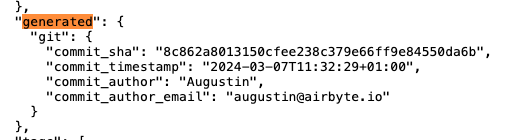 Spun out of #32715 as a stack
- Loading branch information
Showing
21 changed files
with
1,417 additions
and
487 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
35 changes: 35 additions & 0 deletions
35
...e-ci/connectors/metadata_service/lib/metadata_service/models/generated/GeneratedFields.py
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,35 @@ | ||
# generated by datamodel-codegen: | ||
# filename: GeneratedFields.yaml | ||
|
||
from __future__ import annotations | ||
|
||
from datetime import datetime | ||
from typing import Optional | ||
|
||
from pydantic import BaseModel, Extra, Field | ||
|
||
|
||
class GitInfo(BaseModel): | ||
class Config: | ||
extra = Extra.forbid | ||
|
||
commit_sha: Optional[str] = Field( | ||
None, | ||
description="The git commit sha of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI.", | ||
) | ||
commit_timestamp: Optional[datetime] = Field( | ||
None, | ||
description="The git commit timestamp of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI.", | ||
) | ||
commit_author: Optional[str] = Field( | ||
None, | ||
description="The git commit author of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI.", | ||
) | ||
commit_author_email: Optional[str] = Field( | ||
None, | ||
description="The git commit author email of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI.", | ||
) | ||
|
||
|
||
class GeneratedFields(BaseModel): | ||
git: Optional[GitInfo] = None |
31 changes: 31 additions & 0 deletions
31
airbyte-ci/connectors/metadata_service/lib/metadata_service/models/generated/GitInfo.py
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
# generated by datamodel-codegen: | ||
# filename: GitInfo.yaml | ||
|
||
from __future__ import annotations | ||
|
||
from datetime import datetime | ||
from typing import Optional | ||
|
||
from pydantic import BaseModel, Extra, Field | ||
|
||
|
||
class GitInfo(BaseModel): | ||
class Config: | ||
extra = Extra.forbid | ||
|
||
commit_sha: Optional[str] = Field( | ||
None, | ||
description="The git commit sha of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI.", | ||
) | ||
commit_timestamp: Optional[datetime] = Field( | ||
None, | ||
description="The git commit timestamp of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI.", | ||
) | ||
commit_author: Optional[str] = Field( | ||
None, | ||
description="The git commit author of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI.", | ||
) | ||
commit_author_email: Optional[str] = Field( | ||
None, | ||
description="The git commit author email of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI.", | ||
) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -116,3 +116,5 @@ properties: | |
supportsRefreshes: | ||
type: boolean | ||
default: false | ||
generated: | ||
"$ref": GeneratedFields.yaml |
9 changes: 9 additions & 0 deletions
9
airbyte-ci/connectors/metadata_service/lib/metadata_service/models/src/GeneratedFields.yaml
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,9 @@ | ||
--- | ||
"$schema": http://json-schema.org/draft-07/schema# | ||
"$id": https://github.com/airbytehq/airbyte/airbyte-ci/connectors_ci/metadata_service/lib/models/src/GeneratedFields.yaml | ||
title: GeneratedFields | ||
description: Optional schema for fields generated at metadata upload time | ||
type: object | ||
properties: | ||
git: | ||
"$ref": GitInfo.yaml |
21 changes: 21 additions & 0 deletions
21
airbyte-ci/connectors/metadata_service/lib/metadata_service/models/src/GitInfo.yaml
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,21 @@ | ||
--- | ||
"$schema": http://json-schema.org/draft-07/schema# | ||
"$id": https://github.com/airbytehq/airbyte/airbyte-ci/connectors/metadata_service/lib/metadata_service/models/src/GitInfo.yaml | ||
title: GitInfo | ||
description: Information about the author of the last commit that modified this file | ||
type: object | ||
additionalProperties: false | ||
properties: | ||
commit_sha: | ||
type: string | ||
description: The git commit sha of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI. | ||
commit_timestamp: | ||
type: string | ||
format: date-time | ||
description: The git commit timestamp of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI. | ||
commit_author: | ||
type: string | ||
description: The git commit author of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI. | ||
commit_author_email: | ||
type: string | ||
description: The git commit author email of the last commit that modified this file. DO NOT DEFINE THIS FIELD MANUALLY. It will be overwritten by the CI. |
Oops, something went wrong.