-
Notifications
You must be signed in to change notification settings - Fork 772
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat(core): add support for 1..n mediaQuery overrides during printing
When printing developers can now configure how layouts should render by specifying 1..n mediaQuery aliases. This feature allows totally different print outputs without modifying the currently layout. Implement PrintHook service to intercept print mediaQuery activation events. * add fxShow.print and fxHide.print support to show/hide elements during printing * suspend activation changes in MediaMarshaller while print-mode is active * trigger MediaObserver to notify subscribers with print mqAlias(es) * use PrintHook to intercept activation changes in MediaMarshaller while print-mode is active * trigger MediaObserver to notify subscribers with print mqAlias(es) Using the new `printWithBreakpoint` allows developers to specify a breakpoint that should be used to render layouts only during printing. With the configuration below, the breakpoint associated with the **`md`** alias will be used. ```ts FlexLayoutModule.withConfig({ useColumnBasisZero: false, printWithBreakpoints: ['md', 'gt-sm', 'gt-xs'] }) ``` Shown below is the print layout rendered in floating dialog over the normal layout using 'lg' breakpoints. 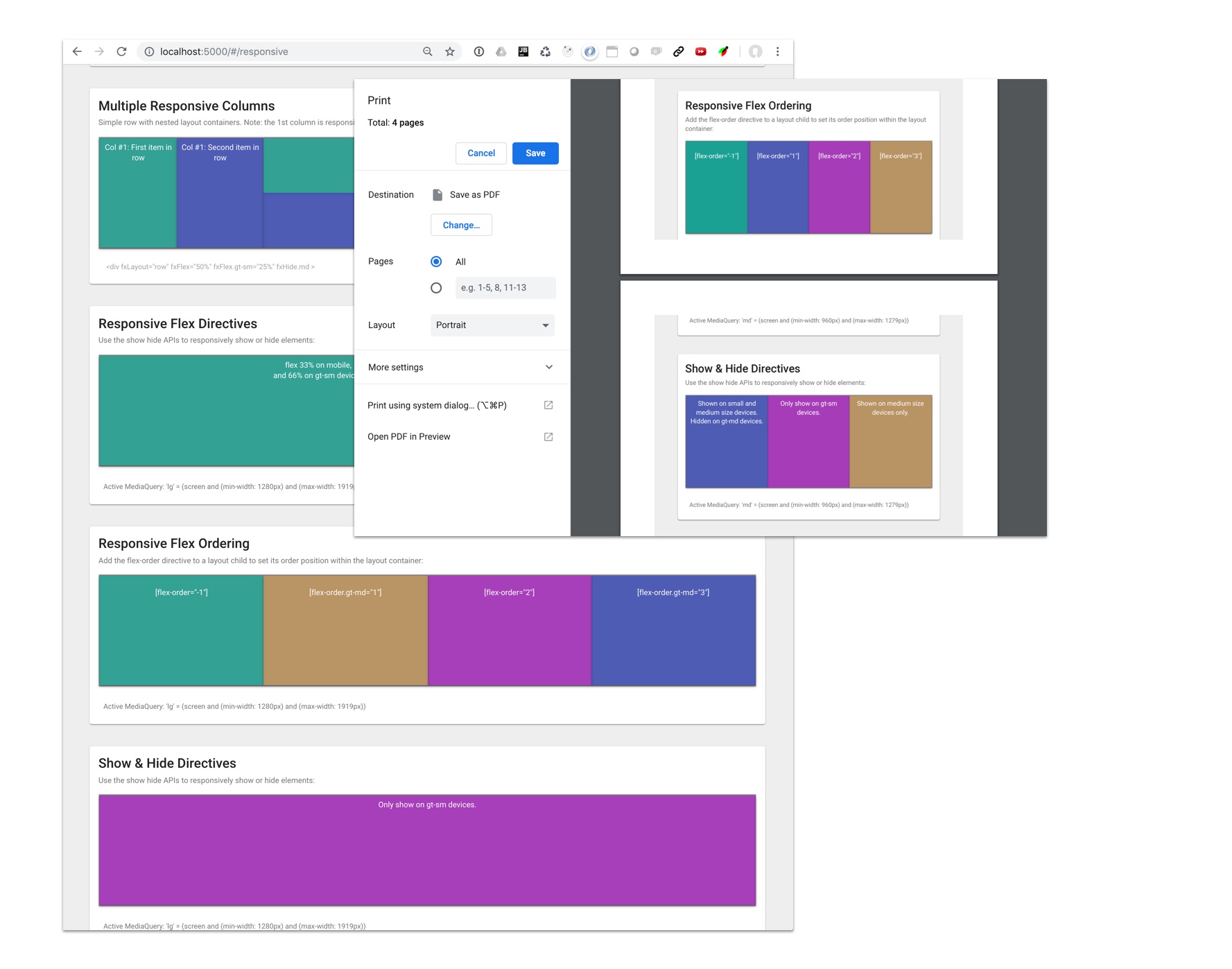 Fixes #603.
- Loading branch information
1 parent
d57b293
commit 9c80f62
Showing
32 changed files
with
1,015 additions
and
329 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,16 @@ | ||
:host { | ||
display: block; | ||
position: absolute; | ||
|
||
width: 100vw; | ||
min-height: 100vh; | ||
top: 0; | ||
left: 0; | ||
right: 0; | ||
bottom: 0; | ||
|
||
div { | ||
width: 100vw; | ||
min-height: 100vh; | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,46 @@ | ||
import {Component, Input} from '@angular/core'; | ||
import {DomSanitizer} from '@angular/platform-browser'; | ||
|
||
@Component({ | ||
selector: 'watermark', | ||
styleUrls: ['watermark.component.scss'], | ||
template: ` | ||
<div [style.background]="backgroundImage"> | ||
</div> | ||
`, | ||
}) | ||
export class WatermarkComponent { | ||
@Input() title = '@angular/layout'; | ||
@Input() message = 'Layout with FlexBox + CSS Grid'; | ||
|
||
constructor(private _sanitizer: DomSanitizer) { | ||
} | ||
|
||
/* tslint:disable:max-line-length */ | ||
get backgroundImage() { | ||
const rawSVG = ` | ||
<svg id="diagonalWatermark" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" | ||
width="100%" height="100%"> | ||
<style type="text/css">text { fill: gray; font-family: Avenir, Arial, Helvetica, sans-serif; | ||
opacity: 0.25; } | ||
</style> | ||
<defs> | ||
<pattern id="titlePattern" patternUnits="userSpaceOnUse" width="400" height="200"> | ||
<text y="30" font-size="40" id="title">${this.title}</text> | ||
</pattern> | ||
<pattern xlink:href="#titlePattern"> | ||
<text y="120" x="200" font-size="30" id="message">${this.message}</text> | ||
</pattern> | ||
<pattern id="combo" xlink:href="#titlePattern" patternTransform="rotate(-45)"> | ||
<use xlink:href="#title"/> | ||
<use xlink:href="#message"/> | ||
</pattern> | ||
</defs> | ||
<rect width="100%" height="100%" fill="url(#combo)"/> | ||
</svg> | ||
`; | ||
const bkgrndImageUrl = `data:image/svg+xml;base64,${window.btoa(rawSVG)}`; | ||
|
||
return this._sanitizer.bypassSecurityTrustStyle(`url('${bkgrndImageUrl}') repeat-y`); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.