-
Notifications
You must be signed in to change notification settings - Fork 8.3k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
## [SIEM] Overview: Recent cases widget
Implements the new `Recent cases` widget on the Overview page. Recent cases shows the last 3 recently created cases, per the following animated gif: 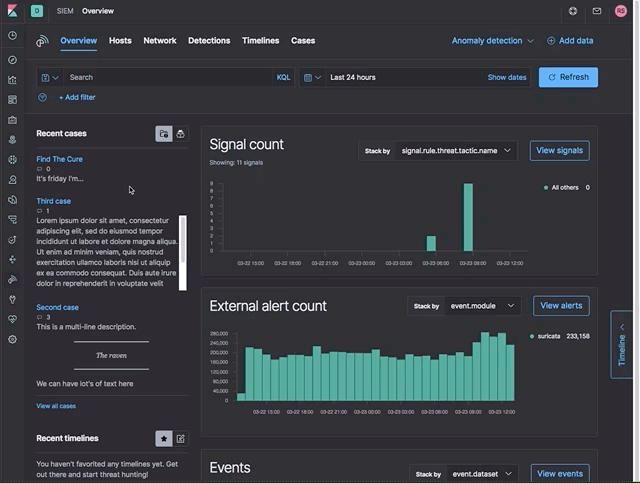 ### Markdown case descriptions Markdown case descriptions are rendered, per the following animated gif: 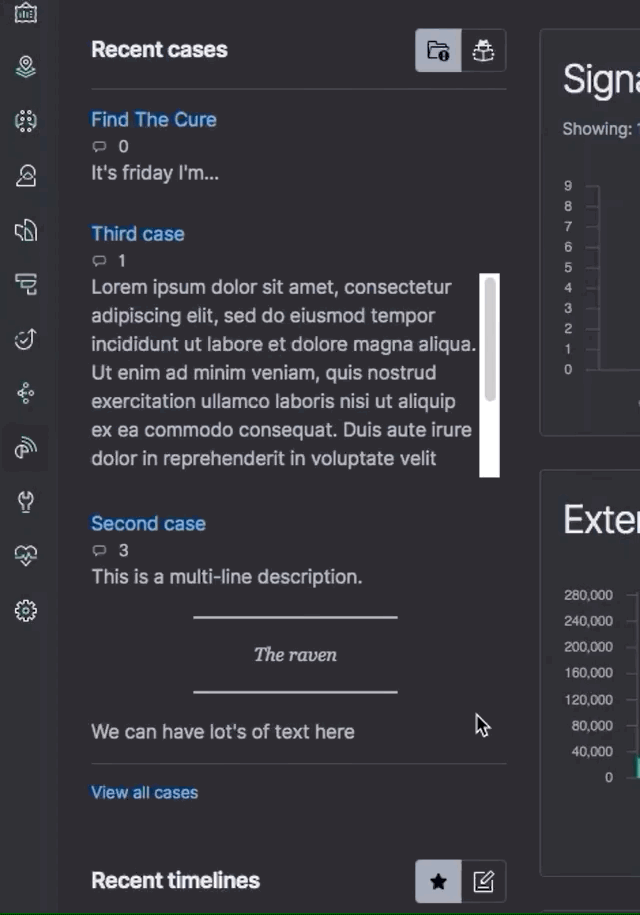 ### My recently reported cases My recently reported cases filters the widget to show only cases created by the logged-in user, per the following animated gif: 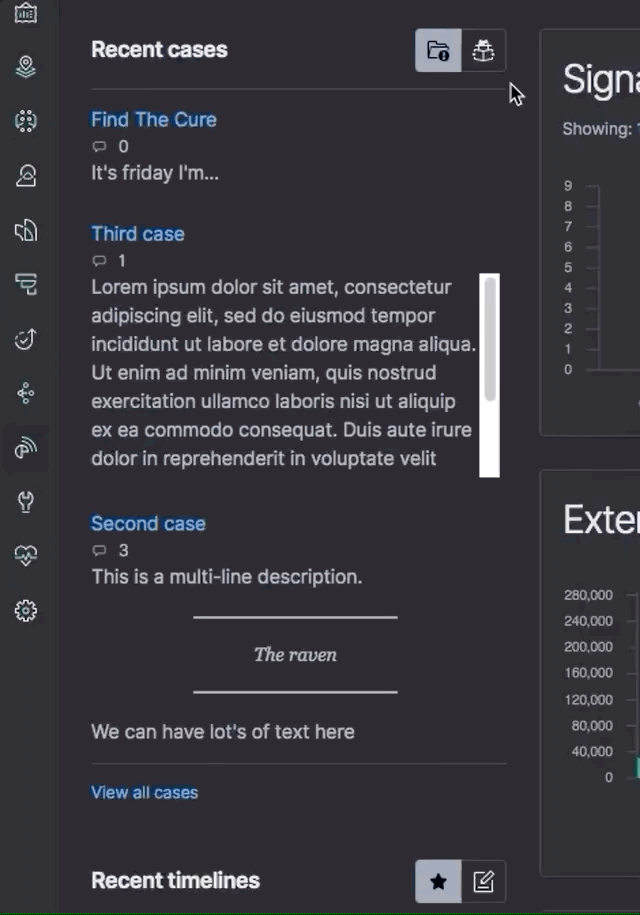 ### No cases state A message welcoming the user to create a case is displayed when no cases exist, per the following screenshot: 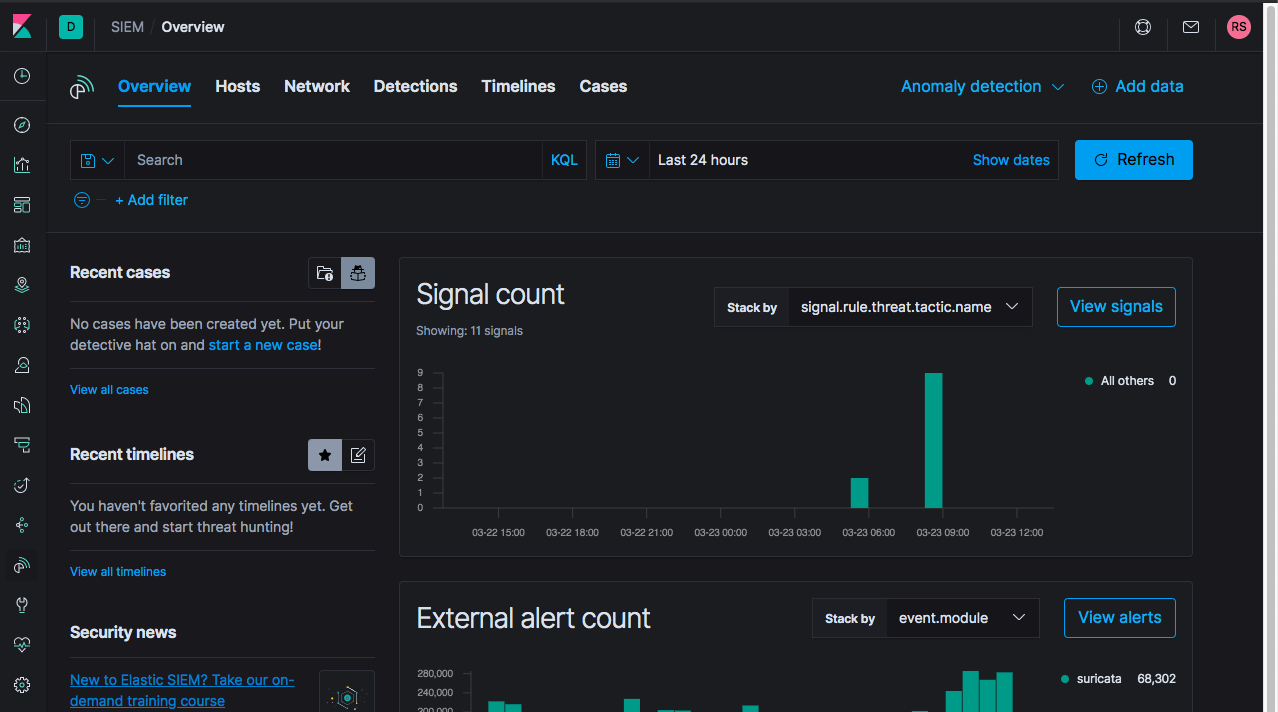 ### Other changes - [x] Case-related links were updated to ensure URL state parameters, e.g. global date selection, carry-over as the user navigates through case views - [x] Recent timelines was updated to only show the last 3 recent timelines (down from 5) - [x] All sidebar widgets have slightly more compact spacing Tested in: * Chrome `80.0.3987.149` * Firefox `74.0` * Safari `13.0.5`
- Loading branch information
1 parent
88d41fa
commit 2f4ec3d
Showing
30 changed files
with
561 additions
and
134 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
54 changes: 54 additions & 0 deletions
54
x-pack/legacy/plugins/siem/public/components/recent_cases/filters/index.tsx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,54 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License; | ||
* you may not use this file except in compliance with the Elastic License. | ||
*/ | ||
|
||
import { EuiButtonGroup, EuiButtonGroupOption } from '@elastic/eui'; | ||
import React, { useMemo } from 'react'; | ||
|
||
import { FilterMode } from '../types'; | ||
|
||
import * as i18n from '../translations'; | ||
|
||
const MY_RECENTLY_REPORTED_ID = 'myRecentlyReported'; | ||
|
||
const toggleButtonIcons: EuiButtonGroupOption[] = [ | ||
{ | ||
id: 'recentlyCreated', | ||
label: i18n.RECENTLY_CREATED_CASES, | ||
iconType: 'folderExclamation', | ||
}, | ||
{ | ||
id: MY_RECENTLY_REPORTED_ID, | ||
label: i18n.MY_RECENTLY_REPORTED_CASES, | ||
iconType: 'reporter', | ||
}, | ||
]; | ||
|
||
export const Filters = React.memo<{ | ||
filterBy: FilterMode; | ||
setFilterBy: (filterBy: FilterMode) => void; | ||
showMyRecentlyReported: boolean; | ||
}>(({ filterBy, setFilterBy, showMyRecentlyReported }) => { | ||
const options = useMemo( | ||
() => | ||
showMyRecentlyReported | ||
? toggleButtonIcons | ||
: toggleButtonIcons.filter(x => x.id !== MY_RECENTLY_REPORTED_ID), | ||
[showMyRecentlyReported] | ||
); | ||
|
||
return ( | ||
<EuiButtonGroup | ||
options={options} | ||
idSelected={filterBy} | ||
onChange={f => { | ||
setFilterBy(f as FilterMode); | ||
}} | ||
isIconOnly | ||
/> | ||
); | ||
}); | ||
|
||
Filters.displayName = 'Filters'; |
75 changes: 75 additions & 0 deletions
75
x-pack/legacy/plugins/siem/public/components/recent_cases/index.tsx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,75 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License; | ||
* you may not use this file except in compliance with the Elastic License. | ||
*/ | ||
|
||
import { EuiHorizontalRule, EuiLink, EuiText } from '@elastic/eui'; | ||
import React, { useEffect, useMemo, useRef } from 'react'; | ||
|
||
import { FilterOptions, QueryParams } from '../../containers/case/types'; | ||
import { DEFAULT_QUERY_PARAMS, useGetCases } from '../../containers/case/use_get_cases'; | ||
import { getCaseUrl } from '../link_to/redirect_to_case'; | ||
import { useGetUrlSearch } from '../navigation/use_get_url_search'; | ||
import { LoadingPlaceholders } from '../page/overview/loading_placeholders'; | ||
import { navTabs } from '../../pages/home/home_navigations'; | ||
|
||
import { NoCases } from './no_cases'; | ||
import { RecentCases } from './recent_cases'; | ||
import * as i18n from './translations'; | ||
|
||
const usePrevious = (value: FilterOptions) => { | ||
const ref = useRef(); | ||
useEffect(() => { | ||
(ref.current as unknown) = value; | ||
}); | ||
return ref.current; | ||
}; | ||
|
||
const MAX_CASES_TO_SHOW = 3; | ||
|
||
const queryParams: QueryParams = { | ||
...DEFAULT_QUERY_PARAMS, | ||
perPage: MAX_CASES_TO_SHOW, | ||
}; | ||
|
||
const StatefulRecentCasesComponent = React.memo( | ||
({ filterOptions }: { filterOptions: FilterOptions }) => { | ||
const previousFilterOptions = usePrevious(filterOptions); | ||
const { data, loading, setFilters } = useGetCases(queryParams); | ||
const isLoadingCases = useMemo( | ||
() => loading.indexOf('cases') > -1 || loading.indexOf('caseUpdate') > -1, | ||
[loading] | ||
); | ||
const search = useGetUrlSearch(navTabs.case); | ||
const allCasesLink = useMemo( | ||
() => <EuiLink href={getCaseUrl(search)}>{i18n.VIEW_ALL_CASES}</EuiLink>, | ||
[search] | ||
); | ||
|
||
useEffect(() => { | ||
if (previousFilterOptions !== undefined && previousFilterOptions !== filterOptions) { | ||
setFilters(filterOptions); | ||
} | ||
}, [previousFilterOptions, filterOptions, setFilters]); | ||
|
||
return ( | ||
<EuiText color="subdued" size="s"> | ||
{isLoadingCases ? ( | ||
<LoadingPlaceholders lines={2} placeholders={3} /> | ||
) : !isLoadingCases && data.cases.length === 0 ? ( | ||
<NoCases /> | ||
) : ( | ||
<RecentCases cases={data.cases} /> | ||
)} | ||
|
||
<EuiHorizontalRule margin="s" /> | ||
<EuiText size="xs">{allCasesLink}</EuiText> | ||
</EuiText> | ||
); | ||
} | ||
); | ||
|
||
StatefulRecentCasesComponent.displayName = 'StatefulRecentCasesComponent'; | ||
|
||
export const StatefulRecentCases = React.memo(StatefulRecentCasesComponent); |
34 changes: 34 additions & 0 deletions
34
x-pack/legacy/plugins/siem/public/components/recent_cases/no_cases/index.tsx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License; | ||
* you may not use this file except in compliance with the Elastic License. | ||
*/ | ||
|
||
import { EuiLink } from '@elastic/eui'; | ||
import React, { useMemo } from 'react'; | ||
|
||
import { getCreateCaseUrl } from '../../link_to/redirect_to_case'; | ||
import { useGetUrlSearch } from '../../navigation/use_get_url_search'; | ||
import { navTabs } from '../../../pages/home/home_navigations'; | ||
|
||
import * as i18n from '../translations'; | ||
|
||
const NoCasesComponent = () => { | ||
const urlSearch = useGetUrlSearch(navTabs.case); | ||
const newCaseLink = useMemo( | ||
() => <EuiLink href={getCreateCaseUrl(urlSearch)}>{` ${i18n.START_A_NEW_CASE}`}</EuiLink>, | ||
[urlSearch] | ||
); | ||
|
||
return ( | ||
<> | ||
<span>{i18n.NO_CASES}</span> | ||
{newCaseLink} | ||
{'!'} | ||
</> | ||
); | ||
}; | ||
|
||
NoCasesComponent.displayName = 'NoCasesComponent'; | ||
|
||
export const NoCases = React.memo(NoCasesComponent); |
60 changes: 60 additions & 0 deletions
60
x-pack/legacy/plugins/siem/public/components/recent_cases/recent_cases.tsx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,60 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License; | ||
* you may not use this file except in compliance with the Elastic License. | ||
*/ | ||
|
||
import { EuiFlexGroup, EuiFlexItem, EuiLink, EuiSpacer, EuiText } from '@elastic/eui'; | ||
import React from 'react'; | ||
import styled from 'styled-components'; | ||
|
||
import { Case } from '../../containers/case/types'; | ||
import { getCaseDetailsUrl } from '../link_to/redirect_to_case'; | ||
import { Markdown } from '../markdown'; | ||
import { useGetUrlSearch } from '../navigation/use_get_url_search'; | ||
import { navTabs } from '../../pages/home/home_navigations'; | ||
import { IconWithCount } from '../recent_timelines/counts'; | ||
|
||
import * as i18n from './translations'; | ||
|
||
const MarkdownContainer = styled.div` | ||
max-height: 150px; | ||
overflow-y: auto; | ||
width: 300px; | ||
`; | ||
|
||
const RecentCasesComponent = ({ cases }: { cases: Case[] }) => { | ||
const search = useGetUrlSearch(navTabs.case); | ||
|
||
return ( | ||
<> | ||
{cases.map((c, i) => ( | ||
<EuiFlexGroup key={c.id} gutterSize="none" justifyContent="spaceBetween"> | ||
<EuiFlexItem grow={false}> | ||
<EuiText size="s"> | ||
<EuiLink href={getCaseDetailsUrl({ id: c.id, search })}>{c.title}</EuiLink> | ||
</EuiText> | ||
|
||
<IconWithCount | ||
count={c.commentIds.length} | ||
icon={'editorComment'} | ||
tooltip={i18n.COMMENTS} | ||
/> | ||
{c.description && c.description.length && ( | ||
<MarkdownContainer> | ||
<EuiText color="subdued" size="xs"> | ||
<Markdown raw={c.description} /> | ||
</EuiText> | ||
</MarkdownContainer> | ||
)} | ||
{i !== cases.length - 1 && <EuiSpacer size="l" />} | ||
</EuiFlexItem> | ||
</EuiFlexGroup> | ||
))} | ||
</> | ||
); | ||
}; | ||
|
||
RecentCasesComponent.displayName = 'RecentCasesComponent'; | ||
|
||
export const RecentCases = React.memo(RecentCasesComponent); |
37 changes: 37 additions & 0 deletions
37
x-pack/legacy/plugins/siem/public/components/recent_cases/translations.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,37 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License; | ||
* you may not use this file except in compliance with the Elastic License. | ||
*/ | ||
|
||
import { i18n } from '@kbn/i18n'; | ||
|
||
export const COMMENTS = i18n.translate('xpack.siem.recentCases.commentsTooltip', { | ||
defaultMessage: 'Comments', | ||
}); | ||
|
||
export const MY_RECENTLY_REPORTED_CASES = i18n.translate( | ||
'xpack.siem.overview.myRecentlyReportedCasesButtonLabel', | ||
{ | ||
defaultMessage: 'My recently reported cases', | ||
} | ||
); | ||
|
||
export const NO_CASES = i18n.translate('xpack.siem.recentCases.noCasesMessage', { | ||
defaultMessage: 'No cases have been created yet. Put your detective hat on and', | ||
}); | ||
|
||
export const RECENTLY_CREATED_CASES = i18n.translate( | ||
'xpack.siem.overview.recentlyCreatedCasesButtonLabel', | ||
{ | ||
defaultMessage: 'Recently created cases', | ||
} | ||
); | ||
|
||
export const START_A_NEW_CASE = i18n.translate('xpack.siem.recentCases.startNewCaseLink', { | ||
defaultMessage: 'start a new case', | ||
}); | ||
|
||
export const VIEW_ALL_CASES = i18n.translate('xpack.siem.recentCases.viewAllCasesLink', { | ||
defaultMessage: 'View all cases', | ||
}); |
7 changes: 7 additions & 0 deletions
7
x-pack/legacy/plugins/siem/public/components/recent_cases/types.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License; | ||
* you may not use this file except in compliance with the Elastic License. | ||
*/ | ||
|
||
export type FilterMode = 'recentlyCreated' | 'myRecentlyReported'; |
Oops, something went wrong.