-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request #9 from hs3city/golangci
Linter, workspace & docs
- Loading branch information
Showing
7 changed files
with
176 additions
and
36 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,55 @@ | ||
name: golangci-lint | ||
on: | ||
push: | ||
branches: [ "main" ] | ||
pull_request: | ||
branches: [ "main" ] | ||
|
||
permissions: | ||
contents: read | ||
# Optional: allow read access to pull request. Use with `only-new-issues` option. | ||
# pull-requests: read | ||
|
||
jobs: | ||
golangci: | ||
name: lint | ||
runs-on: ubuntu-latest | ||
steps: | ||
- uses: actions/checkout@v3 | ||
- uses: actions/setup-go@v4 | ||
with: | ||
go-version: '1.21' | ||
cache: false | ||
- name: golangci-lint | ||
uses: golangci/golangci-lint-action@v3 | ||
with: | ||
# Require: The version of golangci-lint to use. | ||
# When `install-mode` is `binary` (default) the value can be v1.2 or v1.2.3 or `latest` to use the latest version. | ||
# When `install-mode` is `goinstall` the value can be v1.2.3, `latest`, or the hash of a commit. | ||
version: v1.54 | ||
|
||
# Optional: working directory, useful for monorepos | ||
# working-directory: somedir | ||
|
||
# Optional: golangci-lint command line arguments. | ||
# | ||
# Note: By default, the `.golangci.yml` file should be at the root of the repository. | ||
# The location of the configuration file can be changed by using `--config=` | ||
# args: --timeout=30m --config=/my/path/.golangci.yml --issues-exit-code=0 | ||
args: ./cyoa/main ./quiz ./urlshort ./urlshort/main | ||
|
||
# Optional: show only new issues if it's a pull request. The default value is `false`. | ||
# only-new-issues: true | ||
|
||
# Optional: if set to true, then all caching functionality will be completely disabled, | ||
# takes precedence over all other caching options. | ||
# skip-cache: true | ||
|
||
# Optional: if set to true, then the action won't cache or restore ~/go/pkg. | ||
# skip-pkg-cache: true | ||
|
||
# Optional: if set to true, then the action won't cache or restore ~/.cache/go-build. | ||
# skip-build-cache: true | ||
|
||
# Optional: The mode to install golangci-lint. It can be 'binary' or 'goinstall'. | ||
# install-mode: "goinstall" |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1 +1,72 @@ | ||
# goexplorers | ||
|
||
This repository contains various separate modules for [Go](https://go.dev/) self-study coding sessions held at [Hackerspace Tricity](https://github.com/hs3city/). | ||
|
||
In order to have multiple modules in a single repo we use [Go workspaces](https://go.dev/doc/tutorial/workspaces). | ||
|
||
`go.work` file is already created and checked in to the repository, allowing all session participants to have the same developer experience. | ||
|
||
In order to initialize a new Go module in a workspace use the following commands: | ||
|
||
``` | ||
export NEW_MODULE_DIRECTORY=mymodule | ||
mkdir $NEW_MODULE_DIRECTORY | ||
go work use $NEW_MODULE_DIRECTORY | ||
``` | ||
|
||
The last command will modify [go.work](./go.work) file. When adding a new module please also remember to add the directory to [linter configuration](./.github/workflows/golangci-lint.yml#39)<br> | ||
The `args` (`jobs.golangci.steps[golangci-lint].args`) key in the linter config defines a set of module directories to be checked by a suite of linters. | ||
|
||
Having a linter in place allows us to learn best practices and Go idioms while we're learning to walk 🙂 | ||
|
||
|
||
## How to run modules | ||
|
||
### [Quiz](./quiz/) | ||
|
||
``` | ||
go run ./quiz | ||
``` | ||
|
||
|
||
### [Url Shortener](./urlshort/) | ||
|
||
Start the server | ||
``` | ||
go run ./urlshort/... | ||
``` | ||
|
||
Then send a request for a shortened URL to get redirected to it | ||
``` | ||
curl -v localhost:8080/hs3 | ||
``` | ||
|
||
|
||
### [Choose Your Own Adventure](./cyoa/) | ||
|
||
Start the server | ||
``` | ||
cd cyoa/main | ||
go run ./... | ||
``` | ||
|
||
Then point your browser to [localhost:8080/intro](http://localhost:8080/intro) to start the game. | ||
|
||
|
||
## How to run tests for all modules | ||
|
||
``` | ||
go test ./cyoa/... ./quiz/... ./urlshort/... | ||
``` | ||
|
||
From the structure of the command above one can easily guess how to run tests for a single module or how to add more modules.<br> | ||
What if the number of modules is so high that it does not make sense to use that command anymore?<br> | ||
We can use `find` with `maxdepth` flag to select any non-hidden top-level directory, pass it to `sed` to append the dots and finally use `xargs` to invoke the sacred `go` command 💪💪💪 | ||
|
||
``` | ||
find . -maxdepth 1 -type d -not -path '.' -not -path '*/.*' | sed 's|$|/...|' | xargs go test | ||
``` | ||
|
||
Note that the command above depends on the existence of `go.work` file. | ||
|
||
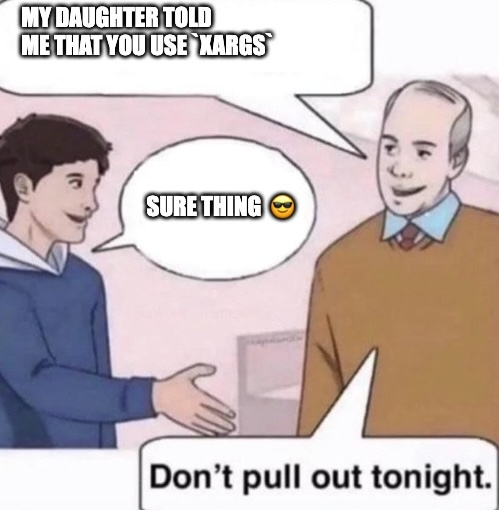 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
go 1.21.3 | ||
|
||
use ( | ||
./cyoa | ||
./quiz | ||
./urlshort | ||
) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters