forked from binary-com/deriv-app
-
Notifications
You must be signed in to change notification settings - Fork 0
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
likhith/migrated hooks folder to ts #58
Merged
jim-deriv
merged 7 commits into
jim-deriv:Jim/77633/components-shared-ts-migration-parent-2
from
likhith-deriv:likhith/76946/migrate-hooks-folder-to-ts
Oct 19, 2022
Merged
Changes from all commits
Commits
Show all changes
7 commits
Select commit
Hold shift + click to select a range
c7d07c2
refactor: :art: migrated hooks folder to ts
likhith-deriv fcf6d25
fix: :recycle: fixed type
likhith-deriv 048ac8c
fix: :recycle: migrated index file\
likhith-deriv e5259a8
fix: :bug: incorrect typing of params
likhith-deriv 62c3ab0
refactor: :recycle: incorporated review comments
likhith-deriv 96ca095
refactor: :recycle: incorporated review comments
likhith-deriv c8cb08d
refactor: :art: added type for callback
likhith-deriv File filter
Filter by extension
Conversations
Failed to load comments.
Loading
Jump to
Jump to file
Failed to load files.
Loading
Diff view
Diff view
There are no files selected for viewing
File renamed without changes.
8 changes: 5 additions & 3 deletions
8
...s/components/src/hooks/use-blockscroll.js → ...s/components/src/hooks/use-blockscroll.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
2 changes: 1 addition & 1 deletion
2
...s/components/src/hooks/use-constructor.js → ...s/components/src/hooks/use-constructor.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
6 changes: 3 additions & 3 deletions
6
...ages/components/src/hooks/use-interval.js → ...ages/components/src/hooks/use-interval.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file was deleted.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
import React, { RefObject } from 'react'; | ||
|
||
export const useOnClickOutside = ( | ||
ref: RefObject<HTMLElement>, | ||
handler: (event: MouseEvent) => void, | ||
validationFn: (event: MouseEvent) => boolean | ||
) => { | ||
React.useEffect(() => { | ||
const listener = (event: MouseEvent) => { | ||
const path = event.composedPath?.()[0] ?? (event as MouseEvent & { path: HTMLElement }).path; //event.path is non-standard and will be deprecated | ||
// When component is isolated (e.g, iframe, shadow DOM) event.target refers to whole container not the component. path[0] is the node that the event originated from, it does not need to walk the array | ||
if ( | ||
ref && | ||
ref.current && | ||
!ref.current.contains(event.target as HTMLElement) && | ||
!ref.current.contains(path as HTMLElement) | ||
) { | ||
if (validationFn && !validationFn(event)) return; | ||
handler(event); | ||
} | ||
}; | ||
|
||
document.addEventListener('mousedown', listener); | ||
|
||
return () => { | ||
document.removeEventListener('mousedown', listener); | ||
}; | ||
}, [ref, handler, validationFn]); | ||
}; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
4 changes: 2 additions & 2 deletions
4
...ages/components/src/hooks/use-previous.js → ...ages/components/src/hooks/use-previous.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,7 +1,7 @@ | ||
import React from 'react'; | ||
|
||
export const usePrevious = value => { | ||
const ref = React.useRef(); | ||
export const usePrevious = <T>(value: T) => { | ||
const ref = React.useRef<T | undefined>(); | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. check please, maybe it is better const ref = React.useRef<T | null>(null); |
||
React.useEffect(() => { | ||
ref.current = value; | ||
}, [value]); | ||
|
10 changes: 6 additions & 4 deletions
10
...es/components/src/hooks/use-safe-state.js → ...es/components/src/hooks/use-safe-state.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
8 changes: 4 additions & 4 deletions
8
...omponents/src/hooks/use-state-callback.js → ...omponents/src/hooks/use-state-callback.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
maybe it better just to use number for types, instead of ReturnType | undefined
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Tried that. But I get the below error
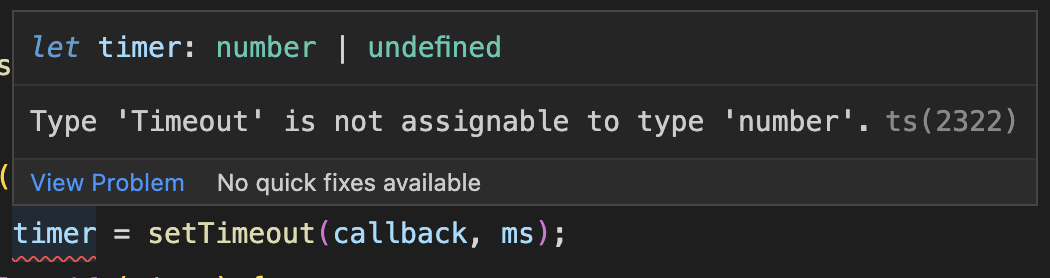