-
-
Notifications
You must be signed in to change notification settings - Fork 10.3k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
How to properly render my drawing (drawlist) in the example "example_sdl_vulkan/main.cpp"? #4644
Comments
Solved, I explored the line ImGui_ImplVulkan_RenderDrawData(draw_data, fd->CommandBuffer); then I found the problem, it changes the scissor. So here is the solution.
|
I guess if your vkPipeline has VK_DYNAMIC_STATE_SCISSOR in its VkDynamicState it will use that left-over state. If you use |
Thanks, the code below is referenced from "Vulkan C++ examples and demos", and it's the default value, so for the moment, I keep it like this but in the future when I need high performance, I will go back to this forum, that's why I always post solution in forums because it will always help me in the future.
Anyway, I will always think about these new lessons. Code simplicity and readability is also important. |
Your solution is to call I suppose we could amend the Vulkan renderer to do that. Not as correct/good as restoring old value (I don't know if we can?) but this would likely be better for a majority of users. |
… / VK_DYNAMIC_STATE_SCISSOR and not calling vkCmdSetViewport() / vkCmdSetScissor(). (#4644)
I made a small change + comment about this:
Not this is technically still not correct but I don't think it is possible to backup/restore that information. |
Thanks a lot. I still have another question related to this thread and I'm going to prepare that, it's "How to get ImGui Windows rectangles? Because I want to make mouse click obstacle of ImGui rectangles from my engine's Window content?". I see hints but it always make zero results. |
The answer to this in the FAQ. Please read the FAQ.
|
I'm back to say thank you and your "change + comment" is already in my real project. The thing that doesn't make me sure is the variable WasActive, it seems to work well without bug but I'm not sure if "does Active means Enabled?". In a 3D software called Blender, active means the very first selected object. I'm not sure if my principle of getting the ImGui windows rectangles is correct because I don't want to be tricked with bugs in the far future.
|
I don't understand why you are using ImGuiWindow* internal data, you should not do that. |
I'm too late, I edited the code, and it was like this before edit:
Do you think it will crash my program in the future? C++ programming is sometimes very abstract in things like this. But it seems currently work without bug. I don't know if it will cause a random time crash in the far future, just trust. |
You should not copy those vectors (use a reference). You should not iterate windows this way unless you have a valid reason. You haven't explained what you are trying to do and why.
|
… / VK_DYNAMIC_STATE_SCISSOR and not calling vkCmdSetViewport() / vkCmdSetScissor(). (ocornut#4644)
Version/Branch of Dear ImGui:
Version: v1.84
Branch:
Back-end/Renderer/Compiler/OS
Back-ends: imgui_impl_sdl.cpp + imgui_impl_vulkan.cpp
Compiler: Visual Studio 2015
Operating System: Windows 10
My Issue/Question:
I'm not a beginner in programming but I'm a beginner with Vulkan because it's too abstract with multi threads theories, I cannot ask this question to the Vulkan forum because it's about ImGui.
How to properly render my drawing in the example "example_sdl_vulkan/main.cpp"? I was migrating from another base called "Vulkan C++ examples and demos" to the official imgui vulkan example, I mean that before my render was perfect without cut glitch not just like in the screenshot.
That text "Hello World!" is not just text, but it's a multiple rectangles with texture.
The reason of the migration is the ImGui example has a perfect mouse behavior, it can drag outside the window, and with dynamic mouse icon change, and it supports keyboard input just like how I typed on the Console in the screenshot.
Screenshot (the glitch is my drawing is cut with unexpected rectangles)
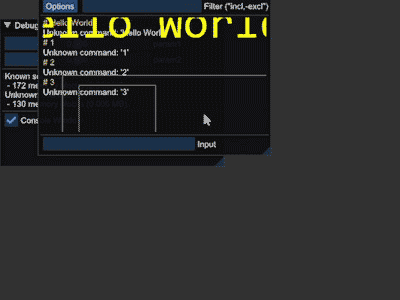
Standalone, minimal, complete and verifiable example:
The function code below is originated from the official Dear ImGui v1.84 >
"examples/example_sdl_vulkan/main.cpp" > FrameRender
There is no modification, but the only change is beetween //==== and //====.
Peek definition of MyEngine::Window::draw
The text was updated successfully, but these errors were encountered: