forked from rust-lang/rust
-
Notifications
You must be signed in to change notification settings - Fork 2
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Upstream sync #38
Merged
Merged
Upstream sync #38
Conversation
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
…haelwoerister Add or improve natvis definitions for common standard library types Natvis definitions are used by Windows debuggers to provide a better experience when inspecting a value for types with natvis definitions. Many of our standard library types and intrinsic Rust types like slices and `str` already have natvis definitions. This PR adds natvis definitions for missing types (like all of the `Atomic*` types) and improves some of the existing ones (such as showing the ref count on `Arc<T>` and `Rc<T>` and showing the borrow state of `RefCell<T>`). I've also added cdb tests to cover these definitions and updated existing tests with the new visualizations. With this PR, the following types now visualize in a much more intuitive way: ### Type: `NonZero{I,U}{8,16,32,64,128,size}`, `Atomic{I,U}{8,16,32,64,size}`, `AtomicBool` and `Wrapping<T>` <details><summary>Example:</summary> ```rust let a_u32 = AtomicU32::new(32i32); ``` ``` 0:000> dx a_u32 a_u32 : 32 [Type: core::sync::atomic::AtomicU32] [<Raw View>] [Type: core::sync::atomic::AtomicU32] ``` </details> ### Type: `Cell<T>` and `UnsafeCell<T>` <details><summary>Example:</summary> ```rust let cell = Cell::new(123u8); let unsafecell = UnsafeCell::new((42u16, 30u16)); ``` ``` 0:000> dx cell cell : 123 [Type: core::cell::Cell<u8>] [<Raw View>] [Type: core::cell::Cell<u8>] 0:000> dx unsafecell unsafecell : (42, 30) [Type: core::cell::UnsafeCell<tuple<u16, u16>>] [<Raw View>] [Type: core::cell::UnsafeCell<tuple<u16, u16>>] [0] : 42 [Type: unsigned short] [1] : 30 [Type: unsigned short] ``` </details> ### Type: `RefCell<T>` <details><summary>Example:</summary> ```rust let refcell = RefCell::new((123u16, 456u32)); ``` ``` 0:000> dx refcell refcell : (123, 456) [Type: core::cell::RefCell<tuple<u16, u32>>] [<Raw View>] [Type: core::cell::RefCell<tuple<u16, u32>>] [Borrow state] : Unborrowed [0] : 123 [Type: unsigned short] [1] : 456 [Type: unsigned int] ``` </details> ### Type: `NonNull<T>` and `Unique<T>` <details><summary>Example:</summary> ```rust let nonnull: NonNull<_> = (&(10, 20)).into(); ``` ``` 0:000> dx nonnull nonnull : NonNull(0x7ff6a5d9c390: (10, 20)) [Type: core::ptr::non_null::NonNull<tuple<i32, i32>>] [<Raw View>] [Type: core::ptr::non_null::NonNull<tuple<i32, i32>>] [0] : 10 [Type: int] [1] : 20 [Type: int] ``` </details> ### Type: `Range<T>`, `RangeFrom<T>`, `RangeInclusive<T>`, `RangeTo<T>` and `RangeToInclusive<T>` <details><summary>Example:</summary> ```rust let range = (1..12); let rangefrom = (9..); let rangeinclusive = (32..=80); let rangeto = (..42); let rangetoinclusive = (..=120); ``` ``` 0:000> dx range range : (1..12) [Type: core::ops::range::Range<i32>] [<Raw View>] [Type: core::ops::range::Range<i32>] 0:000> dx rangefrom rangefrom : (9..) [Type: core::ops::range::RangeFrom<i32>] [<Raw View>] [Type: core::ops::range::RangeFrom<i32>] 0:000> dx rangeinclusive rangeinclusive : (32..=80) [Type: core::ops::range::RangeInclusive<i32>] [<Raw View>] [Type: core::ops::range::RangeInclusive<i32>] 0:000> dx rangeto rangeto : (..42) [Type: core::ops::range::RangeTo<i32>] [<Raw View>] [Type: core::ops::range::RangeTo<i32>] 0:000> dx rangetoinclusive rangetoinclusive : (..=120) [Type: core::ops::range::RangeToInclusive<i32>] [<Raw View>] [Type: core::ops::range::RangeToInclusive<i32>] ``` </details> ### Type: `Duration` <details><summary>Example:</summary> ```rust let duration = Duration::new(5, 12); ``` ``` 0:000> dx duration duration : 5s 12ns [Type: core::time::Duration] [<Raw View>] [Type: core::time::Duration] seconds : 5 [Type: unsigned __int64] nanoseconds : 12 [Type: unsigned int] ``` </details> ### Type: `ManuallyDrop<T>` <details><summary>Example:</summary> ```rust let manuallydrop = ManuallyDrop::new((123, 456)); ``` ``` 0:000> dx manuallydrop manuallydrop : (123, 456) [Type: core::mem::manually_drop::ManuallyDrop<tuple<i32, i32>>] [<Raw View>] [Type: core::mem::manually_drop::ManuallyDrop<tuple<i32, i32>>] [0] : 123 [Type: int] [1] : 456 [Type: int] ``` </details> ### Type: `Pin<T>` <details><summary>Example:</summary> ```rust let mut s = "this".to_string(); let pin = Pin::new(&mut s); ``` ``` 0:000> dx pin pin : Pin(0x11a0ff6f0: "this") [Type: core::pin::Pin<mut alloc::string::String*>] [<Raw View>] [Type: core::pin::Pin<mut alloc::string::String*>] [len] : 4 [Type: unsigned __int64] [capacity] : 4 [Type: unsigned __int64] [chars] ``` </details> ### Type: `Rc<T>` and `Arc<T>` <details><summary>Example:</summary> ```rust let rc = Rc::new(42i8); let rc_weak = Rc::downgrade(&rc); ``` ``` 0:000> dx rc rc : 42 [Type: alloc::rc::Rc<i8>] [<Raw View>] [Type: alloc::rc::Rc<i8>] [Reference count] : 1 [Type: core::cell::Cell<usize>] 0:000> dx rc_weak rc_weak : 42 [Type: alloc::rc::Weak<i8>] [<Raw View>] [Type: alloc::rc::Weak<i8>] ``` </details> r? ```@michaelwoerister``` cc ```@nanguye2496```
…matsakis ExprUseVisitor: Treat ByValue use of Copy types as ImmBorrow r? ```@nikomatsakis```
…matsakis ExprUseVisitor: Treat ByValue use of Copy types as ImmBorrow r? ```@nikomatsakis```
…JohnTitor Correct invariant documentation for `steps_between` Given that the previous example involves stepping forward from A to B, the equivalent example on this line would make most sense as stepping backward from B to A. I should probably add a caveat here that I’m fairly new to Rust, and this is my first contribution to this repo, so it’s very possible that I’ve misunderstood how this is supposed to work (either on a technical level or a social one). If this is the case, please do let me know.
…erister Make --cap-lints and related options leave crate hash alone Closes: rust-lang#87144
…matsakis RFC2229: Use the correct place type Closes rust-lang#87097 The ICE occurred because instead of looking at the type of the place after all the projections are applied, we instead looked at the `base_ty` of the Place to decide whether a discriminant should be read of not. This lead to two issues: 1. the kind of the type is not necessarily `Adt` since we only look at the `base_ty`, it could be instead `Ref` for example 2. if the kind of the type is `Adt` you could still be looking at the wrong variant to make a decision on whether the discriminant should be read or not r? `@nikomatsakis`
…r=notriddle Fix type decl layout "overflow" Before: 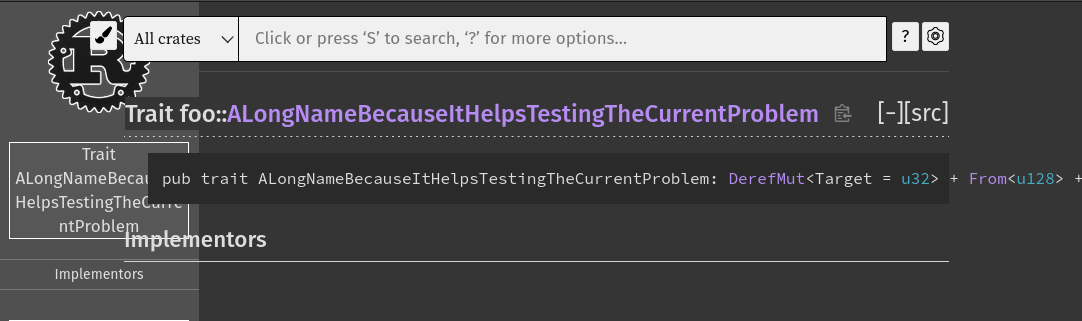 After: 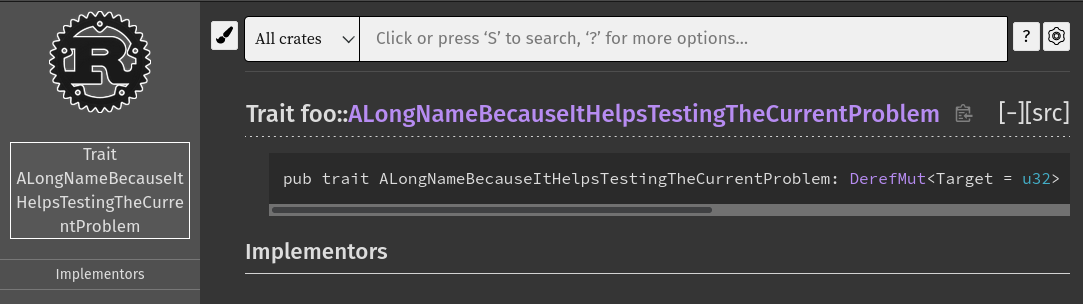 cc ```@SergioBenitez``` r? ```@notriddle```
…le, r=notriddle Fix sidebar display on small devices Part of rust-lang#87059. Instead of hiding the sidebar on small devices, we instead move it out of the viewport so that it remains "visible" to our text only users. Could you confirm it works for you `@ahicks92` and `@DataTriny` please? You can give it a try at [this URL](https://guillaume-gomez.fr/rustdoc-test/test_docs/index.html). r? `@notriddle`
…laumeGomez Rollup of 7 pull requests Successful merges: - rust-lang#86983 (Add or improve natvis definitions for common standard library types) - rust-lang#87069 (ExprUseVisitor: Treat ByValue use of Copy types as ImmBorrow) - rust-lang#87138 (Correct invariant documentation for `steps_between`) - rust-lang#87145 (Make --cap-lints and related options leave crate hash alone) - rust-lang#87161 (RFC2229: Use the correct place type) - rust-lang#87162 (Fix type decl layout "overflow") - rust-lang#87167 (Fix sidebar display on small devices) Failed merges: r? `@ghost` `@rustbot` modify labels: rollup
Remove refs from Pat slices Changes `PatKind::Or(&'hir [&'hir Pat<'hir>])` to `PatKind::Or(&'hir [Pat<'hir>])` and others. This is more consistent with `ExprKind`, saves a little memory, and is a little easier to use.
Remove refs from Pat slices Changes `PatKind::Or(&'hir [&'hir Pat<'hir>])` to `PatKind::Or(&'hir [Pat<'hir>])` and others. This is more consistent with `ExprKind`, saves a little memory, and is a little easier to use.
Fix ICE in redundant_pattern_matching Fixes rust-lang#7410 changelog: Fix ICE in `redundant_pattern_matching` in `no_std` crates.
Fixes rust-lang#87172 Based on rust-lang#87167 (which should be merged first) Preview it at https://notriddle.com/notriddle-rustdoc-test/std/index.html Co-authored-by: Guillaume Gomez <guillaume.gomez@huawei.com>
Make GATs no longer an incomplete feature Blocked on ~rust-lang#84622~, ~rust-lang#82272~, ~rust-lang#76826~ r? `@nikomatsakis`
The previous algorithm was correct for the example given in its documentation, but when the TAIT was declared as a free item instead of an associated item, the generic parameters were the wrong ones.
Loop over all opaque types instead of looking at just the first one with the same DefId This exposed a bug in VecMap and is needed for rust-lang#86410 anyway r? ``@spastorino`` cc ``@nikomatsakis``
…or-local-module, r=estebank Suggest full enum variant for local modules
…nytm Stabilize `[T; N]::map()` This stabilizes the `[T; N]::map()` function, gated by the `array_map` feature. The FCP has [already completed.](rust-lang#75243 (comment)) Closes rust-lang#75243.
Mark `const_trait_impl` as active See [this zulip thread](https://rust-lang.zulipchat.com/#narrow/stream/146212-t-compiler.2Fconst-eval/topic/implementation.20path.20for.20const.20trait.20impls). r? ``@oli-obk``
…d-mobile, r=GuillaumeGomez feat(rustdoc): open sidebar menu when links inside it are focused Fixes rust-lang#87172 Based on rust-lang#87167 (which should be merged first) r? ``@GuillaumeGomez`` Preview it at https://notriddle.com/notriddle-rustdoc-test/std/index.html
…rait-impl, r=notriddle Add GUI test for auto-hide-trait-implementations setting Fixes rust-lang#85592. r? ``@notriddle``
…rgo config not being applied
Rollup of 5 pull requests Successful merges: - rust-lang#81864 (docs: GlobalAlloc: completely replace example with one that works) - rust-lang#87024 (rustdoc: show count of item contents when hidden) - rust-lang#87278 (:arrow_up: rust-analyzer) - rust-lang#87326 (Update cargo) - rust-lang#87346 (Rename force-warns to force-warn) Failed merges: r? `@ghost` `@rustbot` modify labels: rollup
Previously, we would 'forget' that we had `'static` regions in some place during trait evaluation. This lead to us producing `EvaluatedToOkModuloRegions` when we could have produced `EvaluatedToOk`, causing us to perform unnecessary work. This PR preserves `'static` regions when we canonicalize a predicate for `evaluate_obligation`, and when we 'freshen' a predicate during trait evaluation. Thie ensures that evaluating a predicate containing `'static` regions can produce `EvaluatedToOk` (assuming that we don't end up introducing any region dependencies during evaluation). Building off of this improved caching, we use `predicate_must_hold_considering_regions` during fulfillment of projection predicates to see if we can skip performing additional work. We already do this for trait predicates, but doing this for projection predicates lead to mixed performance results without the above caching improvements.
…omatsakis Improve caching during trait evaluation Previously, we would 'forget' that we had `'static` regions in some place during trait evaluation. This lead to us producing `EvaluatedToOkModuloRegions` when we could have produced `EvaluatedToOk`, causing us to perform unnecessary work. This PR preserves `'static` regions when we canonicalize a predicate for `evaluate_obligation`, and when we 'freshen' a predicate during trait evaluation. Thie ensures that evaluating a predicate containing `'static` regions can produce `EvaluatedToOk` (assuming that we don't end up introducing any region dependencies during evaluation). Building off of this improved caching, we use `predicate_must_hold_considering_regions` during fulfillment of projection predicates to see if we can skip performing additional work. We already do this for trait predicates, but doing this for projection predicates lead to mixed performance results without the above caching improvements.
…atsakis When pretty printing, name placeholders as bound regions Split from rust-lang#85499 When we see a placeholder that we are going to print, treat it as a bound var (and add it to a `for<...>`
…nikomatsakis Fix implicit Sized relaxation when attempting to relax other, unsupported trait Fixes rust-lang#87199. Do note that this bug fix causes code like the `ref_arg::<[i32]>(&[5]);` line in the test case in combination with an affected function to no longer compile.
Support HIR wf checking for function signatures During function type-checking, we normalize any associated types in the function signature (argument types + return type), and then create WF obligations for each of the normalized types. The HIR wf code does not currently support this case, so any errors that we get have imprecise spans. This commit extends `ObligationCauseCode::WellFormed` to support recording a function parameter, allowing us to get the corresponding HIR type if an error occurs. Function typechecking is modified to pass this information during signature normalization and WF checking. The resulting code is fairly verbose, due to the fact that we can no longer normalize the entire signature with a single function call. As part of the refactoring, we now perform HIR-based WF checking for several other 'typed items' (statics, consts, and inherent impls). As a result, WF and projection errors in a function signature now have a precise span, which points directly at the responsible type. If a function signature is constructed via a macro, this will allow the error message to point at the code 'most responsible' for the error (e.g. a user-supplied macro argument).
…ywiser Profile incremental compilation hashing fingerprints Adds profiling instrumentation for the hashing of incremental compilation fingerprints per query. This will eventually feed into the `measureme` and `rustc-perf` infrastructure for tracking if computing hashes changes over time. TODOs: * [x] Address the FIXME where we are including node interning in the hash timing. * [ ] Update measureme/summarize to handle this new data: rust-lang/measureme#166 * [ ] ~Update rustc-perf to handle the new data from measureme~ (will be done at a later time) r? `@ghost` cc `@michaelwoerister`
…r=notriddle Don't display <table> in item summary Fixes rust-lang#87231. r? `@notriddle`
Normalize generic_ty before checking if bound is met Fixes rust-lang#81487 r? `@nikomatsakis`
…Gomez rustdoc: Restore --default-theme, etc, by restoring varname escaping In rust-lang#86157 cd0f931 Use Tera templates for rustdoc. dropped the following transformation from the keys of the default settings element's `data-` attribute names: .map(|(k, v)| format!(r#" data-{}="{}""#, k.replace('-', "_"), Escape(v))) The `Escape` part is indeed no longer needed, because Tera does that for us. But the massaging of `-` to `_` is needed, for the (bizarre) reasons explained in the new comments. I have tested that the default theme function works again for me. I have also verified that passing (in shell syntax) '--default-theme="zork&"' escapes the value in the HTML. Closes rust-lang#87263
…=nagisa Allow combining -Cprofile-generate and -Cpanic=unwind when targeting MSVC. The LLVM limitation that previously prevented this has been fixed in LLVM 9 which is older than the oldest LLVM version we currently support. Fixes rust-lang#61002. r? ``@nagisa`` (or anyone else from ``@rust-lang/wg-llvm)``
…on_only_regression_fix, r=cuviper Regression fix to avoid further beta backports: Remove unsound TrustedRandomAccess implementations Removes the implementations that depend on the user-definable trait `Copy`. Only fix regressions to ensure merge in 1.55: Does not modify `vec::IntoIter`. <hr> This PR applies the beta-`1.53` backport rust-lang#86222 (merged as part of rust-lang#86225), a reduced version of rust-lang#85874 that only fixes regressions, to `master` in order to avoid the need for further backports from `1.55` onwards. Beta-`1.54` backport already happened with rust-lang#87136. In case that rust-lang#85874 gets merged quickly (within a week), this PR would be unnecessary. r? `@cuviper`
Update my name/email in .mailmap I should have done this years ago. My name is also scattered across many other rust-lang repos, but none of the other repos I've looked at have a `.mailmap`. Is there any particular reason for this, or any process for updating my name/email everywhere?
…laumeGomez Rollup of 6 pull requests Successful merges: - rust-lang#87270 (Don't display <table> in item summary) - rust-lang#87281 (Normalize generic_ty before checking if bound is met) - rust-lang#87288 (rustdoc: Restore --default-theme, etc, by restoring varname escaping) - rust-lang#87307 (Allow combining -Cprofile-generate and -Cpanic=unwind when targeting MSVC.) - rust-lang#87343 (Regression fix to avoid further beta backports: Remove unsound TrustedRandomAccess implementations) - rust-lang#87357 (Update my name/email in .mailmap) Failed merges: r? `@ghost` `@rustbot` modify labels: rollup
Update cargo 2 commits in 4e143fd131e0c16cefd008456e974236ca54e62e..cebef2951ee69617852844894164b54ed478a7da 2021-07-20 21:55:45 +0000 to 2021-07-22 13:01:52 +0000 - Changes rustc argument from `--force-warns` to `--force-warn` (rust-lang/cargo#9714) - Display registry name instead of registry URL when possible (rust-lang/cargo#9632)
Are there still some tests that don't work? |
Nope, this one should build :) |
Excellent :) bors r+ |
Build succeeded: |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
This merges upstream's master in stages because there were some git issues doing it in one go.